<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Google Map with marker and info window using JavaScript</title> </head> <style> html, body, #map-canvas { height: 600px; margin: 0px; padding: 0px; margin-top:20px; } </style> <body> <script type="text/javascript" src="https://maps.googleapis.com/maps/api/js?v=3.exp"></script> <script type="text/javascript"> function initialize() { /****** Change latitude and longitude here ******/ var myLatlng = new google.maps.LatLng(14.831634, 120.281559); /****** Map Options *******/ var mapOptions = { zoom: 14, center: myLatlng }; var map = new google.maps.Map(document.getElementById('map-canvas'), mapOptions); /****** Info Window Contents *******/ var contentString = '<div id="content">'+ '<div id="siteNotice">'+ '</div>'+ '<h1 id="firstHeading" class="firstHeading">Olongapo City</h1>'+ '<div id="bodyContent">'+ '<div style="float:left; width:20%;"><img src="https://upload.wikimedia.org/wikipedia/commons/thumb/5/50/OlongapoCityjf9265_13.JPG/250px-OlongapoCityjf9265_13.JPG" width="120" height="80"/></div>' + '<div style="float:right; width:80%;margin-top: -6px;"><p>Olongapo, officially the City of Olongapo (Ilocano: Ciudad ti Olongapo; Sambali: Syodad nin Olongapo; Filipino: Lungsod ng Olongapo) and often referred to as Olongapo City, is a highly urbanized city in the Philippines. Located in the province of Zambales but governed independently from the province, it has a population of 233,040 people according to the 2015 census.[4] Along with the town/municipality of Subic (and later, Castillejos as well as the municipalities of Dinalupihan, Hermosa and Morong in Bataan), it comprises Metro Olongapo, one of the twelve metropolitan areas in the Philippines.'+ 'https://en.wikipedia.org/wiki/Olongapo</a> '+ '.</p></div>'+ '</div>'+ '</div>'; var infowindow = new google.maps.InfoWindow({ content: contentString }); /****** Map Marker Options *******/ var marker = new google.maps.Marker({ position: myLatlng, map: map, title: 'Olongapo City (Philippines)' }); /****** Info Window With Click *******/ google.maps.event.addListener(marker, 'click', function() { infowindow.open(map,marker); }); /****** Info Window Without Click *******/ infowindow.open(map,marker); } google.maps.event.addDomListener(window, 'load', initialize); </script> <div class="row"> <div class="col-lg-12"> <div > <div id="map-canvas"></div> </div> </div> </div> </body></html>
article
Friday, April 28, 2017
Google Map with marker and info window using JavaScript
Social popup on page scroll using jQuery and CSS
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Select / Deselect all checkboxes using jQuery</title> </head> <style type="text/css"> /* page text content css */ h1{font-size:45px;text-align:center; text-decoration:underline;} h3{font-size:40px;color:#FA0530} p{font-size:40px;} /* popup css*/ #spopup{ background:#f3f3f3; border-radius:9px; -moz-border-radius:9px; -webkit-border-radius:9px; -moz-box-shadow:inset 0 0 3px #333; -webkit-box-shadow:inset 0 0 3px #333; box-shadow:inner 0 0 3px #333; padding:12px 14px 12px 14px; width:300px; position:fixed; bottom:13px; right:2px; display:none; z-index:90; } </style> <body> <div class="container"> <div class="row"> <div class="col-lg-12"> <div> <h3>Scroll down the page, the social popup would be appear at the right side corner. </h3> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Curabitur ornare facilisis risus venenatis mattis. Fusce convallis vehicula dui sed rhoncus. Donec lorem velit, bibendum ac libero in, feugiat dapibus eros. Donec faucibus enim ac ligula tempus, vitae dignissim felis fermentum. Curabitur lectus orci, tincidunt sit amet ornare ac, scelerisque quis ligula. Nunc iaculis ligula non gravida consequat. Curabitur efficitur placerat odio. Aliquam ut aliquet nunc, in mollis purus. Morbi id orci ut odio suscipit placerat eu suscipit justo. Sed condimentum ligula eu odio bibendum, ac convallis erat finibus.</p> <p>Fusce pellentesque, dui eget auctor luctus, nisl orci aliquam tortor, in blandit mi nulla at urna. Maecenas felis leo, ullamcorper sed vehicula quis, tincidunt non nisi. Praesent iaculis lacus nec commodo facilisis. Sed sagittis ipsum vel cursus venenatis. Mauris dapibus commodo blandit. In tristique diam sem, quis vulputate quam congue sed. Sed cursus nulla a lectus hendrerit ultricies. In vulputate feugiat mauris. Nullam suscipit sed nisl nec facilisis. Nulla luctus justo id semper accumsan. Ut ultricies nisl et cursus convallis. Sed vel arcu ac eros tristique posuere eget ac nibh.</p> <p>Vivamus placerat leo id turpis varius, sit amet vehicula sapien pretium. Etiam quam est, viverra at dui nec, tincidunt volutpat elit. Vestibulum varius velit ut imperdiet tincidunt. Sed venenatis sem sit amet rhoncus hendrerit. Mauris eu accumsan ante. Mauris in accumsan purus. Sed blandit vehicula pharetra. Donec a vehicula elit, sed auctor lectus. Morbi lobortis tempus nulla, sed sollicitudin libero porttitor eu. Pellentesque habitant morbi tristique senectus et netus et malesuada fames ac turpis egestas. Sed convallis arcu vitae sem pulvinar mattis. Duis ac enim dui. Sed et eros pellentesque, bibendum est id, tincidunt mi.</p> <p>Sed ut varius lorem. Duis ac ex sagittis, facilisis eros eu, venenatis quam. Pellentesque sed nunc dui. Praesent scelerisque urna at enim condimentum faucibus. Proin pharetra pellentesque enim, in suscipit neque. Sed sit amet felis nunc. Curabitur eu urna elementum, congue lectus vel, feugiat enim. Nunc commodo euismod metus vitae lobortis. Nullam sit amet ullamcorper justo.</p> </div> <div id="spopup" style="display: none;"> <a style="position:absolute;top:14px;right:10px;color:#555;font-size:10px;font-weight:bold;" href="javascript:void(0);" onClick="return closeSPopup();"><b style="font-size:18px;">X</b></a> <div class="sidebox"> <a class="side-twitter" href="http://twitter.com/kenshin0023"><img src="https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEi7Gr4Wc9bgsUdsyzeXWbNV1r8jMcL0iW5SkjwexRWzWyfsIGZl2FpsVKWWWgWmmMG2KuJikWQLl1OYTubGwc-nhfC4q0PCYI5FRs7_1FuqRWSyXkn0W7G0bB_6aQEXijRZzeDfG0TrEWdE/s400/twitter.gif"/></a><p></p> <a class="side-rss" href="/feeds/posts/default"><img src="https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEgfGHlsDThchOtiET0kwI_1K7zZoq6JH78WftafRUUHRCsZow5zJTFfXBYjVukhZn_5Ljhyl3JCtqEqtmZcYGt-mtZvUV7PmEuLqW95ACwj-WujO4DwY_v2RM9kf_AgufifpuInlS7nxSiM/s400/rss.gif"/></a> </div> </div> </div> </div> </div> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script type="text/javascript" async > $(window).scroll(function(){ if($(document).scrollTop()>=$(document).height()/5) $("#spopup").show("slow");else $("#spopup").hide("slow"); }); function closeSPopup(){ $('#spopup').hide('slow'); } </script> </body></html>
Select / Deselect all checkboxes using jQuery
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Select / Deselect all checkboxes using jQuery</title> </head> <body> <ul class="main"> <li><input type="checkbox" id="select_all" /> Select all</li> <ul> <li><input type="checkbox" class="checkbox" value="1"/>Item 1</li> <li><input type="checkbox" class="checkbox" value="2"/>Item 2</li> <li><input type="checkbox" class="checkbox" value="3"/>Item 3</li> <li><input type="checkbox" class="checkbox" value="4"/>Item 4</li> <li><input type="checkbox" class="checkbox" value="5"/>Item 5</li> </ul> </ul> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script type="text/javascript"> $(document).ready(function(){ $('#select_all').on('click',function(){ if(this.checked){ $('.checkbox').each(function(){ this.checked = true; }); }else{ $('.checkbox').each(function(){ this.checked = false; }); } }); $('.checkbox').on('click',function(){ if($('.checkbox:checked').length == $('.checkbox').length){ $('#select_all').prop('checked',true); }else{ $('#select_all').prop('checked',false); } }); }); </script> </body> </html>
Saturday, April 22, 2017
Smooth scroll to div using jQuery
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Smooth scroll to div using jQuery</title> <style> h1{font-size: 40px;} p{ font-size: 35px;} #top a {padding: 10px;} </style> </head> <body> <div class="container"> <div class="row"> <div class="col-lg-12"> <div id="top"> <a href="#section1">Go Section 1</a>| <a href="#section2">Go Section 2</a>| <a href="#section3">Go Section 3</a>| <a href="#section4">Go Section 4</a>| <a href="#section5">Go Section 5</a> </div> <div id="section1"> <h1>Section 1</h1> <p>Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit</p> </div> <div id="section2"> <h1>Section 2</h1> <a href="#top">BackToTop</a> <p>Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit</p> </div> <div id="section3"> <h1>Section 3</h1> <a href="#top">BackToTop</a> <p>Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit</p> </div> <div id="section4"> <h1>Section 4</h1> <a href="#top">BackToTop</a> <p>Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit</p> </div> <div id="section5"> <h1>Section 5</h1> <a href="#top">BackToTop</a> <p>Neque porro quisquam est qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit</p> </div> </div> </div> </div> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script> $(function() { $('a[href*=#]:not([href=#])').click(function() { var target = $(this.hash); target = target.length ? target : $('[name=' + this.hash.substr(1) +']'); if (target.length) { $('html,body').animate({ scrollTop: target.offset().top }, 1000); return false; } }); }); </script> </body> </html>
Redirect page delay 5 second using JavaScript
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Redirect page delay 5 second using JavaScript</title> <script> function delayRedirect(){ document.getElementById('delayMsg').innerHTML = 'Please wait you\'ll be redirected after <span id="countDown">5</span> seconds....'; var count = 5; setInterval(function(){ count--; document.getElementById('countDown').innerHTML = count; if (count == 0) { window.location = 'http://tutorial101.blogspot.com/'; } },1000); } </script> </head> <body> <div class="container"> <div class="row"> <div class="col-lg-12"> <div class="div-center"> <div id="delayMsg" style="margin-bottom:10px;"></div> <input type="button" onclick="delayRedirect()" value="Click to Redirect"/> </div> </div> </div> </div> </body> </html>
Preview Image before Upload using jQuery
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Preview Image before Upload using jQuery</title> <style type="text/css"> form{float: left;width: 100%;} .div-center img{float: left;margin-top: 20px;} embed{float: left;margin-top: 20px;} </style> </head> <body> <div class="container"> <div class="row"> <div class="col-lg-12"> <div class="div-center"> <form method="post" action="" enctype="multipart/form-data" id="uploadForm"> <input type="file" name="file" id="file" /> </form> </div> </div> </div> </div> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.11.1/jquery.min.js"></script> <script> function filePreview(input) { if (input.files && input.files[0]) { var reader = new FileReader(); reader.onload = function (e) { $('#uploadForm + embed').remove(); $('#uploadForm').after('<embed src="'+e.target.result+'" width="450" height="300">'); } reader.readAsDataURL(input.files[0]); } } $("#file").change(function () { filePreview(this); }); </script> </body> </html>
Friday, April 21, 2017
Create Loader Animation with CSS
<!DOCTYPE HTML> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title> Loader Animation with CSS</title> <style type="text/css"> h2{font-size: 33px;margin-bottom: 10px;} .loader { border-top: 16px solid #4285F4; border-right: 16px solid #EA4335; border-bottom: 16px solid #FBBC05; border-left: 16px solid #34A853; border-radius: 50%; width: 80px; height: 80px; -webkit-animation: rotate 2s linear infinite; animation: rotate 2s linear infinite; } @keyframes rotate { 0% { transform: rotate(0deg); } 100% { transform: rotate(360deg); } } @-webkit-keyframes rotate { 0% { -webkit-transform: rotate(0deg); } 100% { -webkit-transform: rotate(360deg); } } .loader-container { border: 1px solid rgba(255, 255, 255, 0.2); width: 240px; height: 240px; float: left; position: relative; overflow: hidden; -moz-box-sizing: border-box; box-sizing: border-box; } .loader2, .loader2:before, .loader2:after { background: #000000; -webkit-animation: animate 1s infinite ease-in-out; animation: animate 1s infinite ease-in-out; width: 1em; height: 4em; } .loader2 { color: #000000; text-indent: -9999em; margin: 88px auto; position: relative; font-size: 11px; -webkit-transform: translateZ(0); -ms-transform: translateZ(0); transform: translateZ(0); -webkit-animation-delay: -0.16s; animation-delay: -0.16s; } .loader2:before, .loader2:after { position: absolute; top: 0; content: ''; } .loader2:before { left: -1.5em; -webkit-animation-delay: -0.32s; animation-delay: -0.32s; } .loader2:after { left: 1.5em; } @-webkit-keyframes animate { 0%, 80%, 100% { box-shadow: 0 0; height: 4em; } 40% { box-shadow: 0 -2em; height: 5em; } } @keyframes animate { 0%, 80%, 100% { box-shadow: 0 0; height: 4em; } 40% { box-shadow: 0 -2em; height: 5em; } } </style> </head> <body> <div class="container"> <div class="row"> <div class="col-lg-12"> <div class=""> <h2>Loader Style 1</h2> <div class="loader"></div> <h2 style="margin-bottom:0px;">Loader Style 2</h2> <div class="loader-container"> <div class="loader2"></div> </div> </div> </div> </div> </div> </body> </html>
Social Media Floating Sidebar with CSS
<!DOCTYPE HTML> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Social Media Floating Sidebar with CSS</title> <style> body { background-color: #02c54c ;} /* Sticky Social Icons */ .sticky-container{ padding:0px; margin:0px; position:fixed; right:-130px;top:230px; width:210px; z-index: 1100;} .sticky li{list-style-type:none;background-color:#fff;color:#efefef;height:43px;padding:0px;margin:0px 0px 1px 0px; -webkit-transition:all 0.25s ease-in-out;-moz-transition:all 0.25s ease-in-out;-o-transition:all 0.25s ease-in-out; transition:all 0.25s ease-in-out; cursor:pointer;} .sticky li:hover{margin-left:-115px;} .sticky li img{float:left;margin:5px 4px;margin-right:5px;} .sticky li p{padding-top:5px;margin:0px;line-height:16px; font-size:11px;} .sticky li p a{ text-decoration:none; color:#2C3539;} .sticky li p a:hover{text-decoration:underline;} /* Sticky Social Icons */ </style> </head> <body> <div class="container"> <div class="row"> <div class="col-lg-12"> <div class="sticky-container"> <ul class="sticky"> <li> <img src="img/facebook-circle.png" width="32" height="32"> <p><a href="#" target="_blank">Like Us on<br>Facebook</a></p> </li> <li> <img height="32" src="img/twitter-circle.png" width="32" height="32"> <p><a href="#" target="_blank">Follow Us on<br>Twitter</a></p> </li> <li> <img src="img/gplus-circle.png" width="32" height="32"> <p><a href="#" target="_blank">Follow Us on<br>Google+</a></p> </li> <li> <img src="img/linkedin-circle.png" width="32" height="32"> <p><a href="#" target="_blank">Follow Us on<br>LinkedIn</a></p> </li> <li> <img src="img/youtube-circle.png" width="32" height="32"> <p><a href="#" target="_blank">Subscribe on<br>YouYube</a></p> </li> <li> <img src="img/pin-circle.png" width="32" height="32"> <p><a href="#" target="_blank">Follow Us on<br>Pinterest</a></p> </li> </ul> </div> </div> </div> </div> </body> </html>
Thursday, April 13, 2017
Bootstrap Modal Popup Form Submit with Ajax & PHP
//index.php <!-- Latest minified bootstrap css --> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <!-- jQuery library --> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.0/jquery.min.js"></script> <!-- Latest minified bootstrap js --> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> <!-- Button to trigger modal --> <button class="btn btn-success btn-lg" data-toggle="modal" data-target="#modalForm"> Open Contact Form </button> <!-- Modal --> <div class="modal fade" id="modalForm" role="dialog"> <div class="modal-dialog"> <div class="modal-content"> <!-- Modal Header --> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal"> <span aria-hidden="true">×</span> <span class="sr-only">Close</span> </button> <h4 class="modal-title" id="myModalLabel">Contact Form</h4> </div> <!-- Modal Body --> <div class="modal-body"> <p class="statusMsg"></p> <form role="form"> <div class="form-group"> <label for="inputName">Name</label> <input type="text" class="form-control" id="inputName" placeholder="Enter your name"/> </div> <div class="form-group"> <label for="inputEmail">Email</label> <input type="email" class="form-control" id="inputEmail" placeholder="Enter your email"/> </div> <div class="form-group"> <label for="inputMessage">Message</label> <textarea class="form-control" id="inputMessage" placeholder="Enter your message"></textarea> </div> </form> </div> <!-- Modal Footer --> <div class="modal-footer"> <button type="button" class="btn btn-default" data-dismiss="modal">Close</button> <button type="button" class="btn btn-primary submitBtn" onclick="submitContactForm()">SUBMIT</button> </div> </div> </div> </div> <script> function submitContactForm(){ var reg = /^[A-Z0-9._%+-]+@([A-Z0-9-]+\.)+[A-Z]{2,4}$/i; var name = $('#inputName').val(); var email = $('#inputEmail').val(); var message = $('#inputMessage').val(); if(name.trim() == '' ){ alert('Please enter your name.'); $('#inputName').focus(); return false; }else if(email.trim() == '' ){ alert('Please enter your email.'); $('#inputEmail').focus(); return false; }else if(email.trim() != '' && !reg.test(email)){ alert('Please enter valid email.'); $('#inputEmail').focus(); return false; }else if(message.trim() == '' ){ alert('Please enter your message.'); $('#inputMessage').focus(); return false; }else{ $.ajax({ type:'POST', url:'submit_form.php', data:'contactFrmSubmit=1&name='+name+'&email='+email+'&message='+message, beforeSend: function () { $('.submitBtn').attr("disabled","disabled"); $('.modal-body').css('opacity', '.5'); }, success:function(msg){ if(msg == 'ok'){ $('#inputName').val(''); $('#inputEmail').val(''); $('#inputMessage').val(''); $('.statusMsg').html('<span style="color:green;">Thanks for contacting us, we\'ll get back to you soon.</p>'); }else{ $('.statusMsg').html('<span style="color:red;">Some problem occurred, please try again.</span>'); } $('.submitBtn').removeAttr("disabled"); $('.modal-body').css('opacity', ''); } }); } } </script>
<?php //submit_form.php if(isset($_POST['contactFrmSubmit']) && !empty($_POST['name']) && !empty($_POST['email']) && (!filter_var($_POST['email'], FILTER_VALIDATE_EMAIL) === false) && !empty($_POST['message'])){ // Submitted form data $name = $_POST['name']; $email = $_POST['email']; $message= $_POST['message']; /* * Send email to admin */ $to = 'admin@example.com'; $subject= 'Contact Request Submitted'; $htmlContent = ' <h4>Contact request has submitted at tutorial101, details are given below.</h4> <table cellspacing="0" style="width: 300px; height: 200px;"> <tr> <th>Name:</th><td>'.$name.'</td> </tr> <tr style="background-color: #e0e0e0;"> <th>Email:</th><td>'.$email.'</td> </tr> <tr> <th>Message:</th><td>'.$message.'</td> </tr> </table>'; // Set content-type header for sending HTML email $headers = "MIME-Version: 1.0" . "\r\n"; $headers .= "Content-type:text/html;charset=UTF-8" . "\r\n"; // Additional headers $headers .= 'From: tutorial101<sender@example.com>' . "\r\n"; // Send email if(mail($to,$subject,$htmlContent,$headers)){ $status = 'ok'; }else{ $status = 'err'; } // Output status echo $status;die; }
Friday, January 13, 2017
Wordpress Remove Menu admin page
locate wp-admin\includes\plugin.php then add this code
function remove_menus(){ remove_menu_page( 'index.php' ); //Dashboard remove_menu_page( 'jetpack' ); //Jetpack* remove_menu_page( 'edit.php' ); //Posts remove_menu_page( 'upload.php' ); //Media remove_menu_page( 'edit.php?post_type=page' ); //Pages remove_menu_page( 'edit-comments.php' ); //Comments remove_menu_page( 'themes.php' ); //Appearance remove_menu_page( 'plugins.php' ); //Plugins remove_menu_page( 'users.php' ); //Users remove_menu_page( 'tools.php' ); //Tools remove_menu_page( 'options-general.php' ); //Settings } add_action( 'admin_menu', 'remove_menus' );
Saturday, January 7, 2017
CSS target desktop, tablet and mobile?

@media (min-width:320px) { /* smartphones, iPhone, portrait 480x320 phones */ } @media (min-width:481px) { /* portrait e-readers (Nook/Kindle), smaller tablets @ 600 or @ 640 wide. */ } @media (min-width:641px) { /* portrait tablets, portrait iPad, landscape e-readers, landscape 800x480 or 854x480 phones */ } @media (min-width:961px) { /* tablet, landscape iPad, lo-res laptops ands desktops */ } @media (min-width:1025px) { /* big landscape tablets, laptops, and desktops */ } @media (min-width:1281px) { /* hi-res laptops and desktops */ }
Thursday, December 1, 2016
PHPMailer Upload mail Attachment

PHPMailer-master https://github.com/PHPMailer/PHPMailer
<?php $msg = ''; if (array_key_exists('userfile', $_FILES)) { if (preg_match('/\.(doc|docx|xls|xlsx|pdf|jpeg|png)$/i', $_FILES["userfile"]["name"])) //upload { $uploadfile = $_FILES['userfile']['name']; if (move_uploaded_file($_FILES['userfile']['tmp_name'], $uploadfile)) { // This should be somewhere in your include_path require 'PHPMailerAutoload.php'; $mail = new PHPMailer; $mail->setFrom('from@example.com', 'First Last'); $mail->addAddress('tomail@example.com', 'John Doe'); $mail->Subject = 'PHPMailer file sender'; $mail->msgHTML("My message body"); $mail->addAttachment($uploadfile); if (!$mail->send()) { $msg = "Mailer Error: " . $mail->ErrorInfo; } else { $msg = "Message sent!"; } }else { $msg = '<font color=red> ERROR_FAILED_TO_UPLOAD_FILE </font>'; } }else{ $msg = '<font color=red> ERROR_FAILED_TO_UPLOAD_FILE </font>'; } } ?> <!DOCTYPE html> <html> <head> <meta charset="utf-8"/> <title>PHPMailer Upload mail Attachment</title> </head> <body> <?php if (empty($msg)) { ?> <form method="post" enctype="multipart/form-data"> <input type="hidden" name="MAX_FILE_SIZE" value="100000"> Send this file: <input name="userfile" type="file"> <input type="submit" value="Send File"> </form> <?php } else { echo $msg; } ?> </body> </html>
Tuesday, November 29, 2016
Add pagination in wordpress admin in customized plugin
Create folder name admin_menu path wp-content\plugins\admin_menu
Create php file name admin_menu path wp-content\plugins\admin_menu\admin_menu.php
admin_menu.php source code
<?php /** * Plugin Name: Admin Menu * Plugin URI: https://tutorial101.blogspot.com/ * Description: A custom admin menu. * Version: 1.0 * Author: Cairocoders * Author URI: http://tutorial101.blogspot.com/ **/ function theme_options_panel(){ add_menu_page('Theme page title', 'Theme menu', 'manage_options', 'theme-options', 'wps_theme_func'); add_submenu_page('theme-options', 'Settings page title', 'Settings menu label', 'manage_options', 'theme-op-settings', 'wps_theme_func_settings'); add_submenu_page('theme-options', 'FAQ page title', 'FAQ menu label', 'manage_options', 'theme-op-faq', 'wps_theme_func_faq'); add_submenu_page('theme-options', 'My List Table', 'My List Table', 'manage_options', 'my-list-table', 'wps_theme_list_table'); } add_action('admin_menu', 'theme_options_panel'); //function function wps_theme_func(){ echo '<div class="wrap"> <div id="icon-users" class="icon32"></div> <h2>Theme Options</h2> </div>'; } function wps_theme_func_settings(){ echo '<div class="wrap"><div id="icon-options-general" class="icon32"><br></div> <h2>Settings</h2></div>'; } function wps_theme_func_faq(){ echo '<div class="wrap"><div id="icon-options-general" class="icon32"><br></div> <h2>FAQ</h2></div>'; } function wps_theme_list_table(){ echo '<div class="wrap"> <div id="icon-users" class="icon32"></div> <h2>My List Table Test - Pagination</h2> </div>'; global $wpdb; echo '<form method="get" action="" id="posts-filter"> <table class="wp-list-table widefat fixed pages"> <tbody id="the-list"> <thead> <tr> <th class="manage-column column-cb check-column" id="cb" scope="col"></th> <th class="manage-column column-name" scope="col">User ID</th> <th class="manage-column column-name" scope="col">Invoice #</th> <th class="manage-column column-name" scope="col">Date Order</th> <th class="manage-column column-name" scope="col">Paid By</th> <th class="manage-column column-name" scope="col">Action</th> </tr> </thead> <tfoot> <tr> <th class="manage-column column-cb check-column" scope="col"></th> <th class="manage-column column-name" scope="col">User ID</th> <th class="manage-column column-name" scope="col">Invoice #</th> <th class="manage-column column-name" scope="col">Date Order</th> <th class="manage-column column-name" scope="col">Paid By</th> <th class="manage-column column-name" scope="col">Action</th> </tr> </tfoot>'; $pagenum = isset( $_GET['pagenum'] ) ? absint( $_GET['pagenum'] ) : 1; $limit = 3; // number of rows in page $offset = ( $pagenum - 1 ) * $limit; $total = $wpdb->get_var( "SELECT COUNT('id') FROM wp_sdn_userorder" ); $num_of_pages = ceil( $total / $limit ); $sql_usersorder = $wpdb->get_results( "SELECT * FROM wp_sdn_userorder ORDER BY dateorder DESC LIMIT $offset, $limit"); $totalrec = $wpdb->num_rows; $isodd=array('','alternate'); $n=0; foreach ($sql_usersorder as $rows_usersorder) { $n++; $id = $rows_usersorder->id; $user_id = $rows_usersorder->user_id; $course_invoice = $rows_usersorder->course_invoice; $dateorder = $rows_usersorder->dateorder; $paidby = $rows_usersorder->paidby; echo '<tr class="type-page '.$isodd[$n%2].'"> <th class="check-column" scope="row"><center>'.$n.'</center></th> <td class="column-title"><strong><a title="Edit" href="#" class="row-title">'.$user_id.''.rand().'</a></strong> <div class="row-actions"> <span class="edit"><a title="Edit this item" href="#">Edit</a> | </span> <span class="trash"><a href="javascript:delusers('.$id.','.$user_id.')" title="Move this item to the Trash" class="submitdelete">Trash</a> | </span> </div> </td> <td>'.$course_invoice.'</td> <td>'.$dateorder.'</td> <td>'.$paidby.'</td> <td><a href="#">View</a> | <a href="#">Delete</a></td> </tr>'; } echo '</tbody> </table> <div class="tablenav bottom"> <div class="alignleft actions bulkactions">'; $page_links = paginate_links( array( 'base' => add_query_arg( 'pagenum', '%#%' ), 'format' => '', 'prev_text' => __( '«', 'text-domain' ), 'next_text' => __( '»', 'text-domain' ), 'total' => $num_of_pages, 'current' => $pagenum ) ); if ( $page_links ) { echo '<div class="tablenav"><div class="tablenav-pages" style="margin: 1em 0">' . $page_links . '</div></div>'; } echo '</div> <br class="clear"> </div> </form>'; }
Billing Address Same as Shipping Address jQuery
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Billing Address Same as Shipping Address jQuery</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.0/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> <script type="text/javascript"> $(document).ready(function(){ $('#check-address').click(function(){ if ($('#check-address').is(":checked")) { $('#txtfname_billing').val($('#txtfname').val()); $('#txtlname_billing').val($('#txtlname').val()); $('#txtaddress_billing').val($('#txtaddress').val()); $('#txtcity_billing').val($('#txtcity').val()); var country = $('#country option:selected').val(); $('#country_billing option[value=' + country + ']').attr('selected','selected'); } else { //Clear on uncheck $('#txtfname_billing').val(""); $('#txtlname_billing').val(""); $('#txtaddress_billing').val(""); $('#txtcity_billing').val(""); $('#country_billing option[value=""]').attr('selected','selected'); }; }); }); </script> </head> <body> <header class="bg-dark" style="height: 60px; padding: 5px;"> <h3 class="text-light" style="text-align: center;">Billing Address Same as Shipping Address jQuery</h3> </header> <div class="container bg-dark"> <div class="row"> <div class="col-sm-1"></div> <div class="col-sm-2"></div> <div class="col-sm-6 bg-light boxStyle"> <form name="theform" action="" method="post"> <div class="form-group"> <div class="width30 floatL"><label>Firstname</label></div> <div class="width70 floatR"><input type="text" id="txtfname" name="txtfname" placeholder="First Name" class="form-control"></div><br><br> </div> <div class="form-group"> <div class="width30 floatL"><label>Lastname</label></div> <div class="width70 floatR"><input type="text" id="txtlname" name="txtlname" placeholder="Last Name" class="form-control"></div> </div><br> <div class="form-group"> <div class="width30 floatL"><label>Address</label></div> <div class="width70 floatR"><input type="text" id="txtaddress" name="txtaddress" placeholder="Ship to Address" class="form-control"></div> </div><br> <div class="form-group"> <div class="width30 floatL"><label>City</label></div> <div class="width70 floatR"><input type="text" id="txtcity" name="txtcity" placeholder="Ship to City" class="form-control"></div> </div><br> <div class="form-group"> <div class="width30 floatL"><label>Country</label></div> <div class="width70 floatR"><select name="country" size="1" id="country" class="form-control"> <option value="">Select Country..</option> <option value="Philippines">Philippines</option> </select></div> </div><br> <hr/> <p><b>Billing Information <label><input type="checkbox" value="" id="check-address">Same as billing?</label></b></p> <div class="form-group"> <div class="width30 floatL"><label>Firstname</label></div> <div class="width70 floatR"><input type="text" id="txtfname_billing" name="txtfname_billing" placeholder="First Name" class="form-control"> </div><br><br> <div class="form-group"> <div class="width30 floatL"><label>Lastname</label></div> <div class="width70 floatR"><input type="text" id="txtlname_billing" name="txtlname_billing" placeholder="Last Name" class="form-control"></div> </div><br> <div class="form-group"> <div class="width30 floatL"><label>Address</label></div> <div class="width70 floatR"><input type="text" id="txtaddress_billing" name="txtaddress_billing" placeholder="Ship to Address" class="form-control"></div> </div><br> <div class="form-group"> <div class="width30 floatL"><label>City</label></div> <div class="width70 floatR"><input type="text" id="txtcity_billing" name="txtcity_billing" placeholder="Ship to City" class="form-control"></div> </div><br> <div class="form-group"> <div class="width30 floatL"><label>Country</label></div> <div class="width70 floatR"><select name="country_billing" size="1" id="country_billing" class="form-control"> <option value="">Select Country..</option> <option value="Philippines">Philippines</option> </select></div> </div><br> <div class="form-group"> <div class="row"> <div class="floatR"><br/><input class="btn btn-success" type="submit" value="Submit" style="font-weight: bold"></div> </div> </div> </form> </div> <div class="col-sm-1"></div> <div class="col-sm-2"></div> </div> </div> <style> .width30 { width: 30%; } .width70 { width: 70%; } .floatL{ float: left; } .floatR{ float: right; } .boxStyle{ padding: 20px; border-radius: 25px; border-top: 6px solid #dc3545; border-bottom: 6px solid #28a745; } </style> </body> </html>
php Randomize Background Image

<?php $bg = array('bg-01.jpg', 'bg-02.jpg', 'bg-03.jpg', 'bg-04.jpg', 'bg-05.jpg', 'bg-06.jpg', 'bg-07.jpg' ); // array of filenames $i = rand(0, count($bg)-1); // generate random number size of the array $selectedBg = "$bg[$i]"; // set variable equal to which random filename was chosen ?> <style type="text/css"> body{ background: url(images/<?php echo $selectedBg; ?>) no-repeat; } </style>
Saturday, November 26, 2016
Basic Example using SMTP (no authentication) and attachments
require_once('../class.phpmailer.php'); //include("class.smtp.php"); // optional, gets called from within class.phpmailer.php if not already loaded $mail = new PHPMailer(); $body = file_get_contents('contents.html'); $body = eregi_replace("[\]",'',$body); $mail->IsSMTP(); // telling the class to use SMTP $mail->Host = "mail.yourdomain.com"; // SMTP server $mail->SMTPDebug = 2; // enables SMTP debug information (for testing) // 1 = errors and messages // 2 = messages only $mail->SetFrom('name@yourdomain.com', 'First Last'); $mail->AddReplyTo("name@yourdomain.com","First Last"); $mail->Subject = "PHPMailer Test Subject via smtp, basic with no authentication"; $mail->AltBody = "To view the message, please use an HTML compatible email viewer!"; // optional, comment out and test $mail->MsgHTML($body); $address = "whoto@otherdomain.com"; $mail->AddAddress($address, "John Doe"); $mail->AddAttachment("images/phpmailer.gif"); // attachment $mail->AddAttachment("images/phpmailer_mini.gif"); // attachment if(!$mail->Send()) { echo "Mailer Error: " . $mail->ErrorInfo; } else { echo "Message sent!"; }
Saturday, August 13, 2016
Notification Box, Alert Boxes using Jquery CSS
<!DOCTYPE html> <html lang="en"> <head> <title>Notification Box, Alert Boxes using Jquery CSS</title> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js"></script> <style> div.alert-message { display: block; padding: 13px 12px 12px; font-weight: bold; font-size: 14px; color: white; background-color: #2ba6cb; border: 1px solid rgba(0, 0, 0, 0.1); margin-bottom: 12px; -webkit-border-radius: 3px; -moz-border-radius: 3px; -ms-border-radius: 3px; -o-border-radius: 3px; border-radius: 3px; text-shadow: 0 -1px rgba(0, 0, 0, 0.3); position: relative; } div.alert-message .box-icon { display: block; float: left; background-image: url('images/icon.png'); width: 30px; height: 25px; margin-top: -2px; background-position: -8px -8px; } div.alert-message p { margin: 0px; } div.alert-message.success { background-color: #5da423; color: #fff; text-shadow: 0 -1px rgba(0, 0, 0, 0.3); } div.alert-message.success .box-icon { background-position: -48px -8px; } div.alert-message.warning { background-color: #e3b000; color: #fff; text-shadow: 0 -1px rgba(0, 0, 0, 0.3); } div.alert-message.warning .box-icon { background-position: -88px -8px; } div.alert-message.error { background-color: #c60f13; color: #fff; text-shadow: 0 -1px rgba(0, 0, 0, 0.3); } div.alert-message.error .box-icon { background-position: -128px -8px; } div.alert-message a.close { color: #333; position: absolute; right: 4px; top: -1px; font-size: 17px; opacity: 0.2; padding: 4px; } div.alert-message a.close:hover, div.alert-box a.close:focus { opacity: 0.4; } </style> <!-- JavaScript Test Zone --> <script type="text/javascript"> $(function(){ $(".alert-message").delegate("a.close", "click", function(event) { event.preventDefault(); $(this).closest(".alert-message").fadeOut(function(event){ $(this).remove(); }); }); }); </script> </head> <body> <div class="panels"> <div class="panel" id="panel-1"> <div class="alert-message info"> <div class="box-icon"></div> <p>This is an info box<a href="" class="close">×</a> </div> <div class="alert-message success"> <div class="box-icon"></div> <p>This is a success box<a href="" class="close">×</a> </div> <div class="alert-message warning"> <div class="box-icon"></div> <p>This is a warning box<a href="" class="close">×</a> </div> <div class="alert-message error"> <div class="box-icon"></div> <p>This is an alert box<a href="" class="close">×</a> </div> </div> </div> </body> </html>
Friday, August 12, 2016
CodeIgniter - Upload an Image
Copy the following code and store it at application/view/Upload_form.php.
<html> <head> <title>Upload Form</title> </head> <body> <?php echo $error;?> <?php echo form_open_multipart('upload/do_upload');?> <form action = "" method = ""> <input type = "file" name = "userfile" size = "20" /> <br /><br /> <input type = "submit" value = "upload" /> </form> </body> </html>
Copy the code given below and store it at application/view/upload_success.php
<html> <head> <title>Upload Image</title> </head> <body> <h3>Upload an Image Success!</h3> </body> </html>
Copy the code given below and store it at application/controllers/upload.php. Create "uploads" folder at the root of CodeIgniter i.e. at the parent directory of application folder.
<?php class Upload extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form', 'url')); } public function index() { $this->load->view('upload_form', array('error' => ' ' )); } public function do_upload() { $config['upload_path'] = './uploads/'; $config['allowed_types'] = 'gif|jpg|png'; $config['max_size'] = 100; $config['max_width'] = 1024; $config['max_height'] = 768; $this->load->library('upload', $config); if ( ! $this->upload->do_upload('userfile')) { $error = array('error' => $this->upload->display_errors()); $this->load->view('upload_form', $error); } else { $data = array('upload_data' => $this->upload->data()); $this->load->view('upload_success', $data); } } } ?>
Make the following change in the route file in application/config/routes.php and add the following line at the end of file.
$route['upload'] = 'Upload';
Now let us execute this example by visiting the following URL in the browser. Replace the yoursite.com with your URL.
http://yoursite.com/index.php/upload
Download http://bit.ly/2XgSkMN
Django - Template System
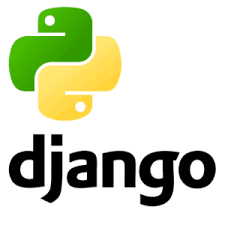
Django makes it possible to separate python and HTML, the python goes in views and HTML goes in templates.
The Render Function
This function takes three parameters −
Request − The initial request.
The path to the template − This is the path relative to the TEMPLATE_DIRS option in the project settings.py variables.
Dictionary of parameters − A dictionary that contains all variables needed in the template. This variable can be created or you can use locals() to pass all local variable declared in the view.
Displaying Variables
A variable looks like this: {{variable}}. The template replaces the variable by the variable sent by the view in the third parameter of the render function.
hello.html
Hello World!!!Today is {{today}}Then our view will change to −
def hello(request): from django.shortcuts import render from django.http import HttpResponse from datetime import datetime def index(request): return render(request, "index.html", {}) def hello(request): myDate = datetime.now() #formatedDate = myDate.strftime("%Y-%m-%d %H:%M:%S") #today = myDate.strftime("%A") today = myDate.strftime("%a") daysOfWeek = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun'] return render(request, "hello.html", {"today" : today, "days_of_week" : daysOfWeek}) #return render(request, "hello.html", {"today" : today})We will now get the following output after accessing the URL/myapp/hello −
Hello World!!!
Today is Sept. 11, 2016
Tag if
Just like in Python you can use if, else and elif in your template −
<html> <body> Hello World!!!<p>Today is {{today}}</p> We are {% if today == 'Sun' %} the Sunday day of month. {% elif today == 30 %} the last day of month. {% else %} I don't know. {%endif%} </body> </html>In this new template, depending on the date of the day, the template will render a certain value.
Tag for
Just like 'if', we have the 'for' tag, that works exactly like in Python. Let's change our hello view to transmit a list to our template −
def hello(request): today = datetime.datetime.now().date() daysOfWeek = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun'] return render(request, "hello.html", {"today" : today, "days_of_week" : daysOfWeek})The template to display that list using {{ for }} −
<html> <body> Hello World!!!<p>Today is {{today}}</p> We are {% if today.day == 1 %} the first day of month. {% elif today == 30 %} the last day of month. {% else %} I don't know. {%endif%} <p> {% for day in days_of_week %} {{day}} </p> {% endfor %} </body> </html>And we should get something like −
Hello World!!!
Today is Sept. 11, 2015
We are I don't know.
Mon
Tue
Wed
Thu
Fri
Sat
Sun
Block and Extend Tags
A template system cannot be complete without template inheritance. Meaning when you are designing your templates, you should have a main template with holes that the child's template will fill according to his own need, like a page might need a special css for the selected tab.
Let’s change the hello.html template to inherit from a main_template.html.
main_template.html
<html> <head> <title> {% block title %}Page Title{% endblock %} </title> </head> <body> {% block content %} Body content {% endblock %} </body> </html>hello.html
{% extends "main_template.html" %} {% block title %}My Hello Page{% endblock %} {% block content %} Hello World!!!Today is {{today}}
We are {% if today.day == 1 %} the first day of month. {% elif today == 30 %} the last day of month. {% else %} I don't know. {%endif%} {% for day in days_of_week %} {{day}}
{% endfor %} {% endblock %}
Cool Notification Messages With CSS3 & jQuery
<!DOCTYPE html> <html> <head> <title>Cool notification messages with CSS3 & Jquery</title> <script src="http://code.jquery.com/jquery-latest.min.js"></script> <script> var myMessages = ['info','warning','error','success']; // define the messages types function hideAllMessages() { var messagesHeights = new Array(); // this array will store height for each for (i=0; i<myMessages.length; i++) { messagesHeights[i] = $('.' + myMessages[i]).outerHeight(); $('.' + myMessages[i]).css('top', -messagesHeights[i]); //move element outside viewport } } function showMessage(type) { $('.'+ type +'-trigger').click(function(){ hideAllMessages(); $('.'+type).animate({top:"0"}, 500); }); } $(document).ready(function(){ // Initially, hide them all hideAllMessages(); // Show message for(var i=0;i<myMessages.length;i++) { showMessage(myMessages[i]); } // When message is clicked, hide it $('.message').click(function(){ $(this).animate({top: -$(this).outerHeight()}, 500); }); }); </script> <style> body { margin: 0; padding: 0; font: 12px Arial, Helvetica, sans-serif; background: #f1f1f1; } .message { -webkit-background-size: 40px 40px; -moz-background-size: 40px 40px; background-size: 40px 40px; background-image: -webkit-gradient(linear, left top, right bottom, color-stop(.25, rgba(255, 255, 255, .05)), color-stop(.25, transparent), color-stop(.5, transparent), color-stop(.5, rgba(255, 255, 255, .05)), color-stop(.75, rgba(255, 255, 255, .05)), color-stop(.75, transparent), to(transparent)); background-image: -webkit-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: -moz-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: -ms-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: -o-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); -moz-box-shadow: inset 0 -1px 0 rgba(255,255,255,.4); -webkit-box-shadow: inset 0 -1px 0 rgba(255,255,255,.4); box-shadow: inset 0 -1px 0 rgba(255,255,255,.4); width: 100%; border: 1px solid; color: #fff; padding: 15px; position: fixed; _position: absolute; text-shadow: 0 1px 0 rgba(0,0,0,.5); -webkit-animation: animate-bg 5s linear infinite; -moz-animation: animate-bg 5s linear infinite; } .info { background-color: #4ea5cd; border-color: #3b8eb5; } .error { background-color: #de4343; border-color: #c43d3d; } .warning { background-color: #eaaf51; border-color: #d99a36; } .success { background-color: #61b832; border-color: #55a12c; } .message h3 { margin: 0 0 5px 0; } .message p { margin: 0; } @-webkit-keyframes animate-bg { from { background-position: 0 0; } to { background-position: -80px 0; } } @-moz-keyframes animate-bg { from { background-position: 0 0; } to { background-position: -80px 0; } } #trigger-list { text-align: center; margin: 100px 0; padding: 0; } #trigger-list li { display: inline-block; *display: inline; zoom: 1; } #trigger-list .trigger { display: inline-block; background: #ddd; border: 1px solid #777; padding: 10px 20px; margin: 0 5px; font: bold 12px Arial, Helvetica; text-decoration: none; color: #333; -moz-border-radius: 3px; -webkit-border-radius: 3px; border-radius: 3px; } #trigger-list .trigger:hover { background: #f5f5f5; } .centered { text-align: center; } .twitter-follow-button { position: relative; top: 7px; } </style> </head> <body> <div class="info message"> <h3>FYI, something just happened!</h3> <p>This is just an info notification message.</p> </div> <div class="error message"> <h3>Ups, an error ocurred</h3> <p>This is just an error notification message.</p> </div> <div class="warning message"> <h3>Wait, I must warn you!</h3> <p>This is just a warning notification message.</p> </div> <div class="success message"> <h3>Congrats, you did it!</h3> <p>This is just a success notification message.</p> </div> <ul id="trigger-list"> <li><a href="#" class="trigger info-trigger">Info</a></li> <li><a href="#" class="trigger error-trigger">Error</a></li> <li><a href="#" class="trigger warning-trigger">Warning</a></li> <li><a href="#" class="trigger success-trigger">Success</a></li> </ul> </body> </html>