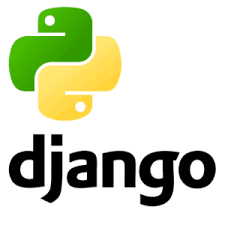
Django makes it possible to separate python and HTML, the python goes in views and HTML goes in templates.
The Render Function
This function takes three parameters −
Request − The initial request.
The path to the template − This is the path relative to the TEMPLATE_DIRS option in the project settings.py variables.
Dictionary of parameters − A dictionary that contains all variables needed in the template. This variable can be created or you can use locals() to pass all local variable declared in the view.
Displaying Variables
A variable looks like this: {{variable}}. The template replaces the variable by the variable sent by the view in the third parameter of the render function.
hello.html
1 | Hello World!!!Today is {{today}} |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 | def hello(request): from django.shortcuts import render from django.http import HttpResponse from datetime import datetime def index(request): return render(request, "index.html" , {}) def hello(request): myDate = datetime.now() #formatedDate = myDate.strftime("%Y-%m-%d %H:%M:%S") #today = myDate.strftime("%A") today = myDate.strftime( "%a" ) daysOfWeek = [ 'Mon' , 'Tue' , 'Wed' , 'Thu' , 'Fri' , 'Sat' , 'Sun' ] return render(request, "hello.html" , { "today" : today, "days_of_week" : daysOfWeek}) #return render(request, "hello.html", {"today" : today}) |
Hello World!!!
Today is Sept. 11, 2016
Tag if
Just like in Python you can use if, else and elif in your template −
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | <html> <body> Hello World!!!<p>Today is {{today}}< / p> We are { % if today = = 'Sun' % } the Sunday day of month. { % elif today = = 30 % } the last day of month. { % else % } I don't know. { % endif % } < / body> < / html> |
Tag for
Just like 'if', we have the 'for' tag, that works exactly like in Python. Let's change our hello view to transmit a list to our template −
1 2 3 4 5 | def hello(request): today = datetime.datetime.now().date() daysOfWeek = [ 'Mon' , 'Tue' , 'Wed' , 'Thu' , 'Fri' , 'Sat' , 'Sun' ] return render(request, "hello.html" , { "today" : today, "days_of_week" : daysOfWeek}) |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | <html> <body> Hello World!!!<p>Today is {{today}}< / p> We are { % if today.day = = 1 % } the first day of month. { % elif today = = 30 % } the last day of month. { % else % } I don't know. { % endif % } <p> { % for day in days_of_week % } {{day}} < / p> { % endfor % } < / body> < / html> |
Hello World!!!
Today is Sept. 11, 2015
We are I don't know.
Mon
Tue
Wed
Thu
Fri
Sat
Sun
Block and Extend Tags
A template system cannot be complete without template inheritance. Meaning when you are designing your templates, you should have a main template with holes that the child's template will fill according to his own need, like a page might need a special css for the selected tab.
Let’s change the hello.html template to inherit from a main_template.html.
main_template.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | <html> <head> <title> { % block title % }Page Title{ % endblock % } < / title> < / head> <body> { % block content % } Body content { % endblock % } < / body> < / html> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 | { % extends "main_template.html" % } { % block title % }My Hello Page{ % endblock % } { % block content % } Hello World!!!Today is {{today}}<br> We are { % if today.day = = 1 % } the first day of month. { % elif today = = 30 % } the last day of month. { % else % } I don't know. { % endif % } { % for day in days_of_week % } {{day}} <br> { % endfor % } { % endblock % } |