<HTML> <HEAD> <SCRIPT language=Javascript> function isNumberKey(evt) { var charCode = (evt.which) ? evt.which : event.keyCode if (charCode > 31 && (charCode < 48 || charCode > 57)) return false; return true; } </SCRIPT> </HEAD> <BODY> <INPUT id="txtChar" onkeypress="return isNumberKey(event)" type="text" name="txtChar"> </BODY> </HTML>
article
Showing posts with label web-development (JavaScript). Show all posts
Showing posts with label web-development (JavaScript). Show all posts
Monday, February 25, 2013
Javascript allow only numbers to be entered in a textbox
Javascript allow only numbers to be entered in a textbox
Sunday, May 13, 2012
Auto iframe’s height using javascript
Auto iframe’s height using javascript
<script type="text/javascript"> function iFrameHeight() { var f = document.getElementById('blockrandom'); f.style.height = '100px' ; var d = (f.contentWindow.document || f.contentDocument) ; var height = Math.max(d.documentElement.scrollHeight, d.body.scrollHeight) ; height += 20; f.style.height = height + 'px' ; f.setAttribute("height", height) ; } </script> <iframe onload="iFrameHeight()" id="blockrandom" name="" src="test.htm" width="100%" height="200" scrolling="no" align="top" frameborder="0" class="wrapper"> No Iframes</iframe>
Sunday, February 26, 2012
JavaScript Date

<html>
<script language="JavaScript">
var mydate=new Date()
var year=mydate.getYear()
if (year < 1000)
year+=1900
var day=mydate.getDay()
var month=mydate.getMonth()
var daym=mydate.getDate()
if (daym<10)
daym="0"+daym
//Arrays for the days of the week, and months of the year
var dayarray=new Array("Sunday","Monday","Tuesday","Wednesday","Thursday","Friday","Saturday")
var montharray=new Array("January","February","March","April","May","June","July","August","September","October","November","December")
//Write out the date.
document.write("<left><small><font color='Black' face='Arial'><b>"+dayarray[day]+", "+montharray[month]+" "+daym+", "+year+"</b></font></small></left>")
</script>
</html>
Thursday, October 20, 2011
Disable Browser Back Button Using Javascript

<HTML>
<HEAD>
<TITLE>Disable Back Button in Browser </TITLE>
<script type="text/javascript">
function disableBackButton()
{
window.history.forward();
}
setTimeout("disableBackButton()", 0);
</script>
</HEAD>
<body onload="disableBackButton()">
<p>This page contains the code to avoid Back button.
</p>
<p>Click here to Goto <a href="noback.html">NoBack Page</a>
</p>
</BODY>
</HTML>
Saturday, August 13, 2011
Sample JavaScript Onload Functions Sample using google map code

<html>
<head>
<title>Google Maps API Sample</title>
<script src="http://maps.google.com/maps?file=api&v=2&sensor=false&key=ABQIAAAAuPsJpk3MBtDpJ4G8cqBnjRRaGTYH6UMl8mADNa0YKuWNNa8VNxQCzVBXTx2DYyXGsTOxpWhvIG7Djw" type="text/javascript"></script>
<script type="text/javascript">
var map = null;
var geocoder = null;
window.onload=initialize; //JavaScript Onload Functions
function initialize() {
if (GBrowserIsCompatible()) {
map = new GMap2(document.getElementById("map_canvas"));
map.setCenter(new GLatLng(37.4419, -122.1419), 13);
geocoder = new GClientGeocoder();
}
var address = '1600 Amphitheatre Pky, Mountain View, CA';
if (geocoder) {
geocoder.getLatLng(
address,
function(point) {
if (!point) {
alert(address + " not found");
} else {
map.setCenter(point, 13);
var marker = new GMarker(point);
map.addOverlay(marker);
marker.openInfoWindowHtml(address);
}
}
);
}
}
</script>
</head>
<body>
<div id="map_canvas" style="width: 500px; height: 300px"></div>
</body>
</html>
Wednesday, July 13, 2011
Create Javascript Popup page

<script language="JavaScript" type="text/JavaScript">
function popup(url,w,h,name,resizable,toolbar,left,top)
{
var features ="location=no,menubars=no,"+"width=550," +"height=550,"+"resizable=yes," +"scrollbars=yes," + "status=yes," + "titlebar=no," +"toolbar="+toolbar+"," +"left="+left+"," +"top="+top;
var win = window.open(url,name,features);
}
</script>
<div>
By clicking Next, you are indicating that you have read and agree to the
<a href="javascript:popup('popuppage.html')" title="Privacy Policy" rel="bookmark">Privacy Policy</a>
</div>
Create a Javascript show hide contents

//css
<style>
.bigbold {
font-weight: bold;
font-size: 25px;
}
.heading
{
background-color: #8FAFBF;
text-align: center;
font-weight: bold;
height: 30px;
}
</style>
//javascript
<script>
function showhideit(id,hid)
{
curstatus = document.getElementById(id).style.display;
if(curstatus == "none")
{
document.getElementById(id).style.display = 'inline';
document.getElementById(hid + '1').innerHTML = ' -';
document.getElementById(hid + '2').innerHTML = '- ';
}
else
{
document.getElementById(id).style.display = 'none';
document.getElementById(hid + '1').innerHTML = ' +';
document.getElementById(hid + '2').innerHTML = '+ ';
}
}
</script>
//HTML
<table align="center" cellpadding="0" cellspacing="0" border="1">
<tr class="heading" style="cursor: pointer;" onclick="showhideit('information','info')">
<td width="100" align="left" class="bigbold" id="info1"> +</td>
<td align="center"><b>CLICK TO SHOW INFORMATION</b></td>
<td width="100" align="right" class="bigbold" id="info2">+ </td>
</tr>
<tr>
<td colspan="3" width="100">
<div style="display:none;" id="information">
PHP Loops
Often when you write code, you want the same block of code to run over and over again in a row. Instead of adding several almost equal lines in a script we can use loops to perform a task like this.
In PHP, we have the following looping statements:
while - loops through a block of code while a specified condition is true
do...while - loops through a block of code once, and then repeats the loop as long as a specified condition is true
for - loops through a block of code a specified number of times
foreach - loops through a block of code for each element in an array
</div>
</td>
</tr>
</table>
Demo
http://dl.dropbox.com/u/7106563/r-ednalan/Show_hide.html
Javascript show hide toggle

<script type="text/javascript">
function toggle(obj){
var el = document.getElementById(obj);
if (el.style.display != 'none') {
el.style.display = 'none';
}
else {
el.style.display = 'inline';
}
}
</script>
<a href="#test1" onclick="toggle('table1');">Toggle 01 </a><br />
<table id="table1" style="display:none;" border="1" cellspacing="0" cellpadding="5">
<tr bgcolor="#CCCCCC">
<td width="100" align="center"><font>PROGRAMME</font></td>
<td width="150" align="center"><font>OBJECTIVES</font></td>
<td width="210" align="center"><font>MODULES</font></td>
</tr>
</table>
<br/>
<a href="#test2" onclick="toggle('table2');">Toggle 02</a><br />
<table id="table2" style="display:none;" border="1" cellspacing="0" cellpadding="5">
<tr bgcolor="#CCCCCC">
<td width="100" align="center"><font>PROGRAMME</font></td>
<td width="150" align="center"><font>OBJECTIVES</font></td>
<td width="210" align="center"><font>MODULES</font></td>
</tr>
</table>
Demo
http://dl.dropbox.com/u/7106563/r-ednalan/show_hide_toggle.html
Javascript One Function to Count and Limit Multiple Form Text Areas

Javascript
<SCRIPT LANGUAGE="JavaScript">
function textCounter(field,cntfield,maxlimit) {
if (field.value.length > maxlimit) // if too long...trim it!
field.value = field.value.substring(0, maxlimit);
// otherwise, update 'characters left' counter
else
cntfield.value = maxlimit - field.value.length;
}
// End -->
</script>
HTML Form
<form name="myForm"
action=""
method="post">
<b>One Function to Count and Limit Multiple Form Text Areas</b><br>
<textarea name="message1" wrap="physical" cols="28" rows="5"
onKeyDown="textCounter(document.myForm.message1,document.myForm.remLen1,125)"
onKeyUp="textCounter(document.myForm.message1,document.myForm.remLen1,125)"></textarea>
<br>
<input readonly type="text" name="remLen1" size="3" maxlength="3" value="125">
characters left
<br>
<textarea name="message2" wrap="physical" cols="28" rows="5"
onKeyDown="textCounter(document.myForm.message2,document.myForm.remLen2,125)"
onKeyUp="textCounter(document.myForm.message2,document.myForm.remLen2,125)"></textarea>
<br>
<input readonly type="text" name="remLen2" size="3" maxlength="3" value="125">
characters left
<br>
<input type="Submit" name="Submit" value="Submit">
<br>
</form>
Friday, July 1, 2011
Create a bubble tooltip using javascript

This demo demonstrates how the balloon tooltip works. Roll your mouse over the links.
CSS Code
<style>
a{
color: #D60808;
text-decoration:none;
}
a:hover{
border-bottom:1px dotted #317082;
color: #307082;
}
#bubble_tooltip{
width:147px;
position:absolute;
display:none;
}
#bubble_tooltip .bubble_top{
background-image: url('images/bubble_top.gif');
background-repeat:no-repeat;
height:16px;
}
#bubble_tooltip .bubble_middle{
background-image: url('images/bubble_middle.gif');
background-repeat:repeat-y;
background-position:bottom left;
padding-left:7px;
padding-right:7px;
}
#bubble_tooltip .bubble_middle span{
position:relative;
top:-8px;
font-family: Trebuchet MS, Lucida Sans Unicode, Arial, sans-serif;
font-size:11px;
}
#bubble_tooltip .bubble_bottom{
background-image: url('images/bubble_bottom.gif');
background-repeat:no-repeat;
background-repeat:no-repeat;
height:44px;
position:relative;
top:-6px;
}
</style>
Javascript code
<script>
function showToolTip(e,text){
if(document.all)e = event;
var obj = document.getElementById('bubble_tooltip');
var obj2 = document.getElementById('bubble_tooltip_content');
obj2.innerHTML = text;
obj.style.display = 'block';
var st = Math.max(document.body.scrollTop,document.documentElement.scrollTop);
if(navigator.userAgent.toLowerCase().indexOf('safari')>=0)st=0;
var leftPos = e.clientX - 100;
if(leftPos<0)leftPos = 0;
obj.style.left = leftPos + 'px';
obj.style.top = e.clientY - obj.offsetHeight -1 + st + 'px';
}
function hideToolTip()
{
document.getElementById('bubble_tooltip').style.display = 'none';
}
</script>
HTML Code
<div id="bubble_tooltip">
<div class="bubble_top"><span></span></div>
<div class="bubble_middle"><span id="bubble_tooltip_content"></span></div>
<div class="bubble_bottom"></div>
</div>
<a href="#" onmouseover="showToolTip(event,'This is the content of the tooltip. This is the content of the tooltip.');return false" onmouseout="hideToolTip()"><h2>Roll over me</h2></a> </p>
Demo
http://dl.dropbox.com/u/3293191/r-ednalan/bubble_tooltip/index.html
Download
http://dl.dropbox.com/u/3293191/r-ednalan/bubble_tooltip/bubble_tooltip.zip
Tuesday, June 28, 2011
IE 5 and 6 png transparent using javascript

<script type="text/javascript" src="js/jquery.js"></script>
this.pngfix = function() {
var ie55 = (navigator.appName == "Microsoft Internet Explorer" && parseInt(navigator.appVersion) == 4 && navigator.appVersion.indexOf("MSIE 5.5") != -1);
var ie6 = (navigator.appName == "Microsoft Internet Explorer" && parseInt(navigator.appVersion) == 4 && navigator.appVersion.indexOf("MSIE 6.0") != -1);
if (jQuery.browser.msie && (ie55 || ie6)) {
$("*").each(function(){
var bgIMG = $(this).css('background-image');
if(bgIMG.indexOf(".png")!=-1){
var iebg = bgIMG.split('url("')[1].split('")')[0];
$(this).css('background-image', 'none');
$(this).get(0).runtimeStyle.filter = "progid:DXImageTransform.Microsoft.AlphaImageLoader(src='" + iebg + "',sizingMethod='crop')";
};
});
};
};
this.init = function() {
pngfix();
};
$(document).ready(function(){
init();
});
Thursday, March 24, 2011
FORM Validators for web developers

LiveValidation is a small open source javascript library for making client-side validation quick, easy, and powerful.
Example Demo
http://livevalidation.com/examples

2. MooTools FormCheck
FormCheck is a class that allows you to perform different tests on form to validate them before submission.
Demo
http://mootools.floor.ch/en/labs/

3. ProtoForm
ProtoForm is an unltra lightweight, unobtrusive cross-browser and very easy to customize form validation + submit with Ajax based on prototype.js framework.
Demo
http://cssrevolt.com/upload/files/protoform/
Monday, February 7, 2011
Draw Charts with Google Visualization API.
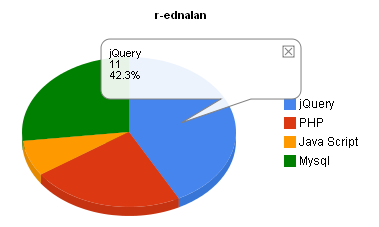
<script type='text/javascript' src='http://www.google.com/jsapi'></script>
<script type="text/javascript">
// Load the Visualization API and the piechart package. Draw Charts with Google Visualization API.
google.load('visualization', '1', {'packages':['piechart']});
// set API loaded.
google.setOnLoadCallback(drawChart);
// draws it.
function drawChart() {
var data = new google.visualization.DataTable();
data.addColumn('string', 'list');
data.addColumn('number', 'Pesentage');
data.addRows(5);
data.setValue(0, 0, 'jQuery');
data.setValue(0, 1, 11);
data.setValue(2, 0, 'PHP');
data.setValue(2, 1,6);
data.setValue(3, 0, 'Java Script');
data.setValue(3, 1, 2);
data.setValue(4, 0, 'Mysql');
data.setValue(4, 1, 7);
var chart = new google.visualization.PieChart(document.getElementById('2'));
chart.draw(data, {width: 400, height: 240, is3D: true, title: 'r-ednalan'});
}
</script>
<div id='2' style="float:left"></div>
Sunday, February 6, 2011
Accordion Menu

The following are Accordion Menu examples with full source code to get you started:
If you want to know more and download the source follow the link below
http://www.dynamicdrive.com/dynamicindex17/ddaccordionmenu.htm
Saturday, February 5, 2011
javascript Accept terms

before submitting the form you must accept term and condition
<script>
var checkobj
function agreesubmit(el){
checkobj=el
if (document.all||document.getElementById){
for (i=0;i<checkobj.form.length;i++){
var tempobj=checkobj.form.elements[i]
if(tempobj.type.toLowerCase()=="submit")
tempobj.disabled=!checkobj.checked
}
}
}
function defaultagree(el){
if (!document.all&&!document.getElementById){
if (window.checkobj&&checkobj.checked)
return true
else{
alert("Please read/accept terms to submit form")
return false
}
}
}
document.forms.agreeform.agreecheck.checked=false
</script>
<form name="agreeform" onSubmit="return defaultagree(this)">
<input name="agreecheck" type="checkbox" onClick="agreesubmit(this)"><b>I agree to the above terms</b><br>
<input type="Submit" value="Submit!" disabled>
</form>
Demo
http://dl.dropbox.com/u/3293191/r-ednalan/accept_term.html
javascript Email Validation

<script type="text/javascript">
var emailfilter=/^\w+[\+\.\w-]*@([\w-]+\.)*\w+[\w-]*\.([a-z]{2,4}|\d+)$/i
function checkmail(e){
var returnval=emailfilter.test(e.value)
if (returnval==false){
alert("Please enter a valid email address.")
e.select()
}
return returnval
}
</script>
<form>
<input name="myemail" type="text" style="width: 270px"> <input type="submit" onClick="return checkmail(this.form.myemail)" value="Submit" />
</form>
Demo
http://dl.dropbox.com/u/3293191/r-ednalan/email_validation.html
Wednesday, June 23, 2010
Scriptaculous ProtoFlow - Prototype

ProtoFlow is a coverflow effect written in Javascript. It uses Prototype and Scriptaculous to do bulk of the work and it uses Reflection.js to do all the image reflections stuff!
If you want to know more and download the source follow the link below
http://www.deensoft.com/lab/protoflow/
Prototype Prototip

Prototip allows you to easily create both simple and complex tooltips using the Prototype javascript framework.
Style: Easy to customize.
Position: Complete control over tooltip positions.
Round: Configurable rounded corners, no PNG images required.
Speech bubble effect!
Works on all modern browsers.
If you want to know more and download the source follow the link below
http://www.nickstakenburg.com/projects/prototip2/
Subscribe to:
Posts (Atom)