from __future__ import division # So that 8/3 will be 2.6666 and not 2 import wx from math import * # So we can evaluate "sqrt(8)" class Calculator(wx.Dialog): '''Main calculator dialog''' def __init__(self): wx.Dialog.__init__(self, None, -1, "Calculator") sizer = wx.BoxSizer(wx.VERTICAL) # Main vertical sizer # ____________v self.display = wx.ComboBox(self, -1) # Current calculation sizer.Add(self.display, 0, wx.EXPAND) # Add to main sizer # [7][8][9][/] # [4][5][6][*] # [1][2][3][-] # [0][.][C][+] gsizer = wx.GridSizer(4, 4) for row in (("7", "8", "9", "/"), ("4", "5", "6", "*"), ("1", "2", "3", "-"), ("0", ".", "C", "+")): for label in row: b = wx.Button(self, -1, label) gsizer.Add(b) self.Bind(wx.EVT_BUTTON, self.OnButton, b) sizer.Add(gsizer, 1, wx.EXPAND) # [ = ] b = wx.Button(self, -1, "=") self.Bind(wx.EVT_BUTTON, self.OnButton, b) sizer.Add(b, 0, wx.EXPAND) self.equal = b # Set sizer and center self.SetSizer(sizer) sizer.Fit(self) self.CenterOnScreen() def OnButton(self, evt): '''Handle button click event''' # Get title of clicked button label = evt.GetEventObject().GetLabel() if label == "=": # Calculate try: compute = self.display.GetValue() # Ignore empty calculation if not compute.strip(): return # Calculate result result = eval(compute) # Add to history self.display.Insert(compute, 0) # Show result self.display.SetValue(str(result)) except Exception, e: wx.LogError(str(e)) return elif label == "C": # Clear self.display.SetValue("") else: # Just add button text to current calculation self.display.SetValue(self.display.GetValue() + label) self.equal.SetFocus() # Set the [=] button in focus if __name__ == "__main__": # Run the application app = wx.App() dlg = Calculator() dlg.ShowModal() dlg.Destroy()
article
Sunday, May 27, 2018
wxpython Calculator
wxpython Calculator
wxpython layout wx.GridSizer
wxpython layout wx.GridSizer
The wx.GridSizer lays out widgets in two dimensional table. Each cell within the table has the same size.
wx.GridSizer(int rows=1, int cols=0, int vgap=0, int hgap=0)
The wx.GridSizer lays out widgets in two dimensional table. Each cell within the table has the same size.
wx.GridSizer(int rows=1, int cols=0, int vgap=0, int hgap=0)
import wx class Example(wx.Frame): def __init__(self, parent, title): super(Example, self).__init__(parent, title=title, size=(300, 250)) self.InitUI() self.Centre() self.Show() def InitUI(self): menubar = wx.MenuBar() fileMenu = wx.Menu() menubar.Append(fileMenu, '&File') self.SetMenuBar(menubar) vbox = wx.BoxSizer(wx.VERTICAL) self.display = wx.TextCtrl(self, style=wx.TE_RIGHT) vbox.Add(self.display, flag=wx.EXPAND|wx.TOP|wx.BOTTOM, border=4) gs = wx.GridSizer(5, 4, 5, 5) gs.AddMany( [(wx.Button(self, label='Cls'), 0, wx.EXPAND), (wx.Button(self, label='Bck'), 0, wx.EXPAND), (wx.StaticText(self), wx.EXPAND), (wx.Button(self, label='Close'), 0, wx.EXPAND), (wx.Button(self, label='7'), 0, wx.EXPAND), (wx.Button(self, label='8'), 0, wx.EXPAND), (wx.Button(self, label='9'), 0, wx.EXPAND), (wx.Button(self, label='/'), 0, wx.EXPAND), (wx.Button(self, label='4'), 0, wx.EXPAND), (wx.Button(self, label='5'), 0, wx.EXPAND), (wx.Button(self, label='6'), 0, wx.EXPAND), (wx.Button(self, label='*'), 0, wx.EXPAND), (wx.Button(self, label='1'), 0, wx.EXPAND), (wx.Button(self, label='2'), 0, wx.EXPAND), (wx.Button(self, label='3'), 0, wx.EXPAND), (wx.Button(self, label='-'), 0, wx.EXPAND), (wx.Button(self, label='0'), 0, wx.EXPAND), (wx.Button(self, label='.'), 0, wx.EXPAND), (wx.Button(self, label='='), 0, wx.EXPAND), (wx.Button(self, label='+'), 0, wx.EXPAND) ]) vbox.Add(gs, proportion=1, flag=wx.EXPAND) self.SetSizer(vbox) if __name__ == '__main__': app = wx.App() Example(None, title='Calculator') app.MainLoop()
wxpython layout Absolute Positioning
wxpython layout Absolute Positioning
import wx class Example(wx.Frame): def __init__(self, parent, title): super(Example, self).__init__(parent, title=title, size=(260, 180)) self.InitUI() self.Centre() self.Show() def InitUI(self): menubar = wx.MenuBar() filem = wx.Menu() editm = wx.Menu() helpm = wx.Menu() menubar.Append(filem, '&File') menubar.Append(editm, '&Edit') menubar.Append(helpm, '&Help') self.SetMenuBar(menubar) #wx.TextCtrl(panel, pos=(3, 3), size=(250, 150)) wx.TextCtrl(self) #auto reize if __name__ == '__main__': app = wx.App() Example(None, title='Absolute Positioning') app.MainLoop()
wxpython enable and disable toolbar buttons
wxpython enable and disable toolbar buttons
import wx class Example(wx.Frame): def __init__(self, *args, **kwargs): super(Example, self).__init__(*args, **kwargs) self.InitUI() def InitUI(self): self.count = 5 self.toolbar = self.CreateToolBar() tundo = self.toolbar.AddLabelTool(wx.ID_UNDO, '', wx.Bitmap('img/blogger.png')) tredo = self.toolbar.AddLabelTool(wx.ID_REDO, '', wx.Bitmap('img/youtube.png')) self.toolbar.EnableTool(wx.ID_REDO, False) self.toolbar.AddSeparator() texit = self.toolbar.AddLabelTool(wx.ID_EXIT, '', wx.Bitmap('img/icon_shortcut_tickets.png')) self.toolbar.Realize() self.Bind(wx.EVT_TOOL, self.OnQuit, texit) self.Bind(wx.EVT_TOOL, self.OnUndo, tundo) self.Bind(wx.EVT_TOOL, self.OnRedo, tredo) self.SetSize((250, 200)) self.SetTitle('Undo redo') self.Centre() self.Show(True) def OnUndo(self, e): if self.count > 1 and self.count <= 5: self.count = self.count - 1 if self.count == 1: self.toolbar.EnableTool(wx.ID_UNDO, False) if self.count == 4: self.toolbar.EnableTool(wx.ID_REDO, True) def OnRedo(self, e): if self.count < 5 and self.count >= 1: self.count = self.count + 1 if self.count == 5: self.toolbar.EnableTool(wx.ID_REDO, False) if self.count == 2: self.toolbar.EnableTool(wx.ID_UNDO, True) def OnQuit(self, e): self.Close() def main(): ex = wx.App() Example(None) ex.MainLoop() if __name__ == '__main__': main()
wxpython create more than one toolbars example
wxpython create more than one toolbars example
import wx class Example(wx.Frame): def __init__(self, *args, **kwargs): super(Example, self).__init__(*args, **kwargs) self.InitUI() def InitUI(self): vbox = wx.BoxSizer(wx.VERTICAL) #create two horizontal toolbars toolbar1 = wx.ToolBar(self) toolbar1.AddLabelTool(wx.ID_ANY, '', wx.Bitmap('img/icon_shortcut_contacts.png')) toolbar1.AddLabelTool(wx.ID_ANY, '', wx.Bitmap('img/icon_shortcut_looong.png')) toolbar1.AddLabelTool(wx.ID_ANY, '', wx.Bitmap('img/icon_shortcut_sales.png')) toolbar1.Realize() toolbar2 = wx.ToolBar(self) qtool = toolbar2.AddLabelTool(wx.ID_EXIT, '', wx.Bitmap('img/icon_shortcut_tickets.png')) toolbar2.Realize() vbox.Add(toolbar1, 0, wx.EXPAND) vbox.Add(toolbar2, 0, wx.EXPAND) self.Bind(wx.EVT_TOOL, self.OnQuit, qtool) self.SetSizer(vbox) self.SetSize((300, 250)) self.SetTitle('Toolbars') self.Centre() self.Show(True) def OnQuit(self, e): self.Close() def main(): ex = wx.App() Example(None) ex.MainLoop() if __name__ == '__main__': main()
Ajax Jquery and php Page Loading
Ajax Jquery and php Page Loading
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Ajax Jquery and php Page Loading</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.0/jquery.min.js"></script> <script type="text/javascript"> $(document).ready(function(){ $('.super').click(function(){ $('#container').fadeOut(); var a = $(this).attr('id'); $.post("ajax_page.php?id="+a, { }, function(response){ setTimeout("finishAjax('container', '"+escape(response)+"')", 400); }); }); }); function finishAjax(id, response){ $('#'+id).html(unescape(response)); $('#'+id).fadeIn(); } </script> </head> <body> <div style="float:left"> <ul class="menus"> <li><input type="button" name="test" class="super red button" id="1" value="Home" /></li> <li><input type="button" name="test" class="super buy button" id="2" value="About" /></li> <li><input type="button" name="test" class="super green button" id="3" value="Services" /></li> </ul> </div> <div id="container"> <?php include('dbcon.php'); $query = $conn->query("SELECT * FROM descriptions where page_type = 1 order by id desc"); while ($row = $query ->fetch_object()) { $heading=$row->heading; $text=$row->text; ?> <label><?php echo $heading;?></label> <br /> <p><?php echo $text;?></p> <?php } ?> </div> <style> ul.menus{ list-style:none; margin:10px 0 0 0; } ul.menus li input { display:block; padding:8px 8px 8px 8px; text-decoration:none; color:#ddd; font-size:22px; text-shadow:1px 1px 1px #000; margin:5px 10px; background-color:#1f1f1f; border:1px solid #222; -moz-box-shadow:0px 0px 10px #000; -webkit-box-shadow:0px 0px 10px #000; box-shadow:0px 0px 10px #000; background-repeat:no-repeat; background-position:5px 50%; opacity:0.9; outline:none; width:120px; } ul.menus li input:hover{ color:#fff; border:1px solid #303030; background-color:#212121; opacity:1.0; text-shadow:0px 0px 1px #fff; } #container{ -moz-border-radius: 6px; -webkit-border-radius: 6px; -moz-box-shadow: 0 1px 3px rgba(0,0,0,0.6); -webkit-box-shadow: 0 1px 3px rgba(0,0,0,0.6); padding:10px 20px 20px 20px; text-align:justify; font-size:14px; font-family:Arial, Helvetica, sans-serif; text-shadow: 0 -1px 1px rgba(0,0,0,0.25); height:300px; float:left; width:400px; } #container label{ font-size:24px; color:#336699; font-weight:bolder; } </style> </body> </html>
//ajax_page.php <?php include("dbcon.php"); $getid = $_REQUEST['id']; $sql = 'SELECT * FROM descriptions where page_type = "'.$getid.'" order by id desc'; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { while($row = mysqli_fetch_assoc($result)) { $heading = $row["heading"]; $text = $row["text"]; echo "<label>$heading</label><br />"; echo "<p>$text</label></p>"; } } else { echo "0 results"; } mysqli_close($conn); ?>
//dbcon.php <?php $conn = new mysqli('localhost','root','','testingdb'); if ($conn->connect_error) { die('Error : ('. $conn->connect_errno .') '. $conn->connect_error); } ?>
AJAX Pagination using jQuery, PHP and Msqli
AJAX Pagination using jQuery, PHP and Msqli
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>AJAX Pagination using jQuery, PHP and Msqli</title> <link href="http://netdna.bootstrapcdn.com/bootstrap/3.0.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css"> <script src="http://netdna.bootstrapcdn.com/bootstrap/3.0.0/js/bootstrap.min.js"></script> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.0/jquery.min.js"></script> <script type="text/javascript"> $(document).ready(function(){ function showLoader(){ $('.search-background').fadeIn(200); } function hideLoader(){ $('.search-background').fadeOut(200); }; $("#paging_button li").click(function(){ showLoader(); $("#paging_button li").css({'background-color' : ''}); $(this).css({'background-color' : '#006699'}); $("#content").load("ajaxpagenation.php?page=" + this.id, hideLoader); return false; }); $("#1").css({'background-color' : '#006699'}); showLoader(); $("#content").load("ajaxpagenation.php?page=1", hideLoader); }); </script> <style type="text/css"> .trash { color:rgb(209, 91, 71); } .flag { color:rgb(248, 148, 6); } .panel-body { padding:0px; } .panel-footer .pagination { margin: 0; } .panel .glyphicon,.list-group-item .glyphicon { margin-right:5px; } .list-group { margin-bottom:0px; } </style> </head> <body> <?php include("dbcon.php"); $per_page = 10; $sql="SELECT * FROM country "; if ($result=mysqli_query($conn,$sql)){ $count=mysqli_num_rows($result); $pages = ceil($count/$per_page); mysqli_free_result($result); } ?> <div class="container"> <div class="row"> <div class="col-md-12" style="padding:30px;"> <div class="panel panel-primary"> <div class="panel-heading"> <div class="search-background"><img src="img/loader.gif" alt="" />Loading..</div> <span class="glyphicon glyphicon-list"></span>AJAX Pagination using jQuery, PHP and Mysqli </div> <div class="panel-body"> <ul class="list-group"> <div id="content"></div> </ul> </div> <div class="panel-footer"> <div class="row"> <div class="col-md-12"> <div id="paging_button" > <ul class="pagination pagination-sm pull-right"> <?php //Show page links for($i=1; $i<=$pages; $i++) { ?> <li id="<?php echo $i; ?>"><a href="#"><?php echo $i; ?></a></li> <?php }?> </ul> </div> </div> </div> </div> </div> </div> </div> </div> </body> </html>
//ajaxpagenation.php <?php include("dbcon.php"); $per_page = 10; $sqlc="SELECT * FROM country"; if ($rsdc=mysqli_query($conn,$sqlc)){ $cols=mysqli_num_rows($rsdc); } $page = $_REQUEST['page']; $start = ($page-1)*10; $sql = $conn->query("SELECT * FROM country ORDER BY id limit $start,10"); while($rows = $sql->fetch_assoc()) { ?> <li class="list-group-item"> <img src="img/philflag.png" width="35" height="30"> <span><b><?php echo $rows['country'];?></b></span> <div class="pull-right action-buttons"> <a href="#"><span class="glyphicon glyphicon-pencil"></span></a> <a href="#" class="trash"><span class="glyphicon glyphicon-trash"></span></a> <a href="#" class="flag"><span class="glyphicon glyphicon-flag"></span></a> </div> </li> <?php } ?>
//dbcon.php <?php $conn = new mysqli('localhost','root','','testingdb'); if ($conn->connect_error) { die('Error : ('. $conn->connect_errno .') '. $conn->connect_error); } ?>
Friday, May 11, 2018
Password Strength Checker jQuery

<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>Password Strength Checker jQuery</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> </head> <body> <style type="text/css"> #passwordTest { width: 400px; background: #F0F0F0; padding: 20px; border: 1px solid #DDD; border-radius: 4px; } #passwordTest input[type="password"]{ width: 97.5%; height: 25px; margin-bottom: 5px; border: 1px solid #DDD; border-radius: 4px; line-height: 25px; padding-left: 5px; font-size: 25px; color: #829CBD; } #pass-info{ width: 97.5%; height: 25px; border: 1px solid #DDD; border-radius: 4px; color: #829CBD; text-align: center; font: 12px/25px Arial, Helvetica, sans-serif; } #pass-info.weakpass{ border: 1px solid #FF9191; background: #FFC7C7; color: #94546E; text-shadow: 1px 1px 1px #FFF; } #pass-info.stillweakpass { border: 1px solid #FBB; background: #FDD; color: #945870; text-shadow: 1px 1px 1px #FFF; } #pass-info.goodpass { border: 1px solid #C4EEC8; background: #E4FFE4; color: #51926E; text-shadow: 1px 1px 1px #FFF; } #pass-info.strongpass { border: 1px solid #6ED66E; background: #79F079; color: #348F34; text-shadow: 1px 1px 1px #FFF; } #pass-info.vrystrongpass { border: 1px solid #379137; background: #48B448; color: #CDFFCD; text-shadow: 1px 1px 1px #296429; } </style> <script type="text/javascript"> $(document).ready(function() { var password1 = $('#password1'); //id of first password field var password2 = $('#password2'); //id of second password field var passwordsInfo = $('#pass-info'); //id of indicator element passwordStrengthCheck(password1,password2,passwordsInfo); //call password check function }); function passwordStrengthCheck(password1, password2, passwordsInfo) { //Must contain 5 characters or more var WeakPass = /(?=.{5,}).*/; //Must contain lower case letters and at least one digit. var MediumPass = /^(?=\S*?[a-z])(?=\S*?[0-9])\S{5,}$/; //Must contain at least one upper case letter, one lower case letter and one digit. var StrongPass = /^(?=\S*?[A-Z])(?=\S*?[a-z])(?=\S*?[0-9])\S{5,}$/; //Must contain at least one upper case letter, one lower case letter and one digit. var VryStrongPass = /^(?=\S*?[A-Z])(?=\S*?[a-z])(?=\S*?[0-9])(?=\S*?[^\w\*])\S{5,}$/; $(password1).on('keyup', function(e) { if(VryStrongPass.test(password1.val())) { passwordsInfo.removeClass().addClass('vrystrongpass').html("Very Strong! (Awesome, please don't forget your pass now!)"); } else if(StrongPass.test(password1.val())) { passwordsInfo.removeClass().addClass('strongpass').html("Strong! (Enter special chars to make even stronger"); } else if(MediumPass.test(password1.val())) { passwordsInfo.removeClass().addClass('goodpass').html("Good! (Enter uppercase letter to make strong)"); } else if(WeakPass.test(password1.val())) { passwordsInfo.removeClass().addClass('stillweakpass').html("Still Weak! (Enter digits to make good password)"); } else { passwordsInfo.removeClass().addClass('weakpass').html("Very Weak! (Must be 5 or more chars)"); } }); $(password2).on('keyup', function(e) { if(password1.val() !== password2.val()) { passwordsInfo.removeClass().addClass('weakpass').html("Passwords do not match!"); }else{ passwordsInfo.removeClass().addClass('goodpass').html("Passwords match!"); } }); } </script> <h2>Password Strength Checker jQuery</h2> <form action="" method="post" id="passwordTest"> <div><input type="password" id="password1" /></div> <div><input type="password" id="password2" /></div> <div id="pass-info"></div> </form> </body> </html>
Wednesday, May 9, 2018
how to enabling mod rewrite in xampp
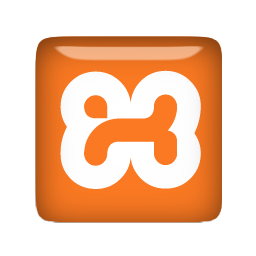
1. go to the directory of installation
2. Find the line which contains
3. #LoadModule rewrite_module modules/mod_rewrite.so
uncomment this(should be):
4. LoadModule rewrite_module modules/mod_rewrite.so
5. Also find AllowOverride None
6. Should be: AllowOverride All
Friday, May 4, 2018
PHP curl not installed - Getting cURL to Work in XAMPP
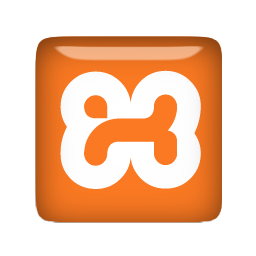
1. Go to xampp install directory. xampp\php\php.ini
2. Go to php.ini in php directory.
3. Open php.ini and find curl, uncomment the first find you see. extension=php_curl.dll
4. Save and close file.
5. restart xampp
6. Done
Sunday, April 29, 2018
How to Load or Read XML file using PHP
How to Load or Read XML file using PHP
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>How to Load or Read XML file using PHP</title> </head> <body> <?php //Read Specific XML Elements $xmldata = simplexml_load_file("xml/employee.xml") or die("Failed to load"); echo $xmldata->employee[0]->firstname . "<br/>"; echo $xmldata->employee[1]->firstname . "<br/>"; echo $xmldata->employee[2]->firstname; ?><br/><br/> <?php //Read XML Elements In A Loop $xmldata = simplexml_load_file("xml/employee.xml") or die("Failed to load"); foreach($xmldata->children() as $empl) { echo $empl->firstname . ", "; echo $empl->lastname . ", "; echo $empl->designation . ", "; echo $empl->salary . "<br/>"; } ?> <br/><br/> <?php $xml = simplexml_load_file('xml/employee.xml'); echo '<h2>Employees Listing</h2>'; $list = $xml->employee; for ($i = 0; $i < count($list); $i++) { echo 'Name: ' . $list[$i]->firstname . '<br>'; echo 'Salary: ' . $list[$i]->salary . '<br>'; echo 'Position: ' . $list[$i]->designation . '<br><br>'; } ?> </body> </html>
//employee.xml <?xml version="1.0"?> <company> <employee> <firstname>Emma</firstname> <lastname>Cruise</lastname> <designation>MD</designation> <salary>500000</salary> </employee> <employee> <firstname>Olivia</firstname> <lastname>Horne</lastname> <designation>CEO</designation> <salary>250000</salary> </employee> <employee> <firstname>Isabella</firstname> <lastname>Sophia</lastname> <designation>Finance Manager</designation> <salary>250000</salary> </employee> </company>
Saturday, April 28, 2018
Highlight table row record on hover - jQuery
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Highlight table row record on hover - jQuery</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.0/jquery.min.js"></script> </head> <body> <h1>Highlight table row record on hover - jQuery</h1> <table border="1"> <tr><th>No</th><th>Name</th><th>Age</th><th>Salary</th></tr> <tr><td>1</td><td>Kenshin Himura</td><td>28</td><td>$100,000</td></tr> <tr><td>1</td><td>Kenshin Himura</td><td>28</td><td>$100,000</td></tr> </table> <script type="text/javascript"> $("tr").not(':first').hover( function () { $(this).css("background","#195A72"); }, function () { $(this).css("background",""); } ); </script> </body> </html>
Sunday, April 15, 2018
HTML5 Inline Edit with jQuery Ajax, PHP & MYSQL
HTML5 Inline Edit with jQuery Ajax, PHP & MYSQLi
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>HTML5 Inline Content Editing with jQuery, PHP & MYSQL</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script> $(function(){ var message_status = $("#status"); $("td[contenteditable=true]").blur(function(){ var field_userid = $(this).attr("id"); var value = $(this).text(); var string = value; $.post("ajax_inlineupdate.php", { string: string,field_userid: field_userid}, function(data) { if(data != '') { message_status.show(); message_status.text(data); //hide the message setTimeout(function(){message_status.hide()},1000); } }); }); }); </script> <style> table.zebra-style { font-family:"Lucida Sans Unicode", "Lucida Grande", Sans-Serif; text-align:left; border:1px solid #ccc; margin-bottom:25px; width:50% } table.zebra-style th { color: #444; font-size: 13px; font-weight: normal; padding: 10px 8px; } table.zebra-style td { color: #777; padding: 8px; font-size:13px; } table.zebra-style tr.odd { background:#f2f2f2; } body { background:#fafafa; } .container { width: 800px; border: 1px solid #C4CDE0; border-radius: 2px; margin: 0 auto; height: 1300px; background:#fff; padding-left:10px; } #status { padding:10px; background:#88C4FF; color:#000; font-weight:bold; font-size:12px; margin-bottom:10px; display:none; width:90%; } </style> </head> <body> <h1>HTML5 Inline Edit with jQuery Ajax, PHP & MYSQLi</h1> <div id="status"></div> <table class="table zebra-style"> <thead> <tr> <th>#</th> <th>First Name</th> <th>Last Name</th> <th>City</th> </tr> </thead> <tbody> <tr class="odd"> <td>1</td> <td id="f:1" contenteditable="true">Michael</td> <td id="l:1" contenteditable="true">Holz</td> <td id="c:1" contenteditable="true">Olongapo City</td> </tr> <tr> <td>2</td> <td id="f:2" contenteditable="true">Paula</td> <td id="l:2" contenteditable="true">Wilson</td> <td id="c:2" contenteditable="true">California</td> </tr> <tr class="odd"> <td>3</td> <td id="f:3" contenteditable="true">Antonio</td> <td id="l:3" contenteditable="true">Moreno</td> <td id="c:3" contenteditable="true">Olongapo City</td> </tr> </tbody> </table> </body> </html>
//ajax_inlineupdate.php <?php include"dbcon.php"; $string = $_POST['string']; $field_userid = $_POST['field_userid']; if ($string==''){ echo "<p class='btn btn-info' align='center'>Please Insert field</p>"; }else{ $strings = $field_userid; $fields = $strings[0]; if ($fields=='f') { $setrs = "fname='$string'"; }elseif ($fields=='l') { $setrs = "lname='$string'"; }elseif ($fields=='c') { $setrs = "city='$string'"; }else{$setrs = "";} $getid = substr($field_userid, 2); $sql = "UPDATE user SET $setrs WHERE id = '$getid' "; if ($conn->query($sql) === TRUE) { echo "Record updated successfully"; } else { echo "Error updating record: " . $conn->error; } } ?>
//dbcon.php <?php $conn = new mysqli('localhost','root','','testingdb'); if ($conn->connect_error) { die('Error : ('. $conn->connect_errno .') '. $conn->connect_error); } ?>
Friday, April 13, 2018
PHP Function create SEO URL Friendly

strtlower make string lowercase preg_replace remove all unwanted character spaces and dashes
<?php function seo_url($string, $seperator='-') { $string = strtolower($string); $string = preg_replace("/[^a-z0-9_\s-]/", $seperator, $string); $string = preg_replace("/[\s-]+/", " ", $string); $string = preg_replace("/[\s_]/", $seperator, $string); return $string; } $teststring = "PHP Function create SEO URL Friendly"; $seofrieldy = seo_url($teststring); echo "www.tutorial.com/$seofrieldy/"; ?>
Saturday, March 31, 2018
Dynamic Select Box using Jquery Ajax php mysql

How to create a dynamic select box using jquery ajax php and mysql
Database
CREATE TABLE `rme_city` ( `idarea` int(11) NOT NULL, `city` varchar(255) NOT NULL, `contryid` varchar(200) NOT NULL DEFAULT '' ) ENGINE=MyISAM DEFAULT CHARSET=latin1; INSERT INTO `rme_city` (`idarea`, `city`, `contryid`) VALUES (1, 'Oakland', '4'), (2, 'San Diego', '4'), (21, 'Birmingham', '1'), (22, 'Montgomery', '1'); ALTER TABLE `rme_city` MODIFY `idarea` int(11) NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=23;
index.php
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Dynamic Select Box using Jquery Ajax php mysqli</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.0/jquery.min.js"></script> <script type="text/javascript"> $(document).ready(function() { $(".country").change(function() { var id=$(this).val(); var dataString = 'id='+ id; $.ajax ({ type: "POST", url: "city.php", data: dataString, cache: false, success: function(html) { $(".city").html(html); } }); }); }); </script> </head> <body> <p>State : <select name="country" class="country select" style="width:250px" > <option value="1">Alabama</option> <option value="2">Alaska</option> <option value="3">Arizona</option> <option value="4" selected>California</option> <option value="5">Colorado</option> <option value="6">Connecticut</option> <option value="7">Florida</option> <option value="8">Georgia</option> </select></p> <p>City : <select name="city" style="width:250px" class="city select"> <option value="" selected="selected" >Please Select Your Area</option> </select></p> </body> </html>
city.php
<?php $mysqli = new mysqli('localhost','root','','testingdb'); if ($mysqli->connect_error) { die('Error : ('. $mysqli->connect_errno .') '. $mysqli->connect_error); } if($_POST['id']) { $id=$_POST['id']; $results = $mysqli->query("SELECT * FROM rme_city where contryid='$id' ORDER BY city ASC"); while($row = $results->fetch_assoc()) { $id=$row['idarea']; $data=$row['city']; echo '<option value="'.$id.'">'.$data.'</option>'; } } ?>
Saturday, March 24, 2018
Multiple Image Upload Php

how to upload multiple files using a single form
<?php if (isset($_POST['Submit'])){ while(list($key,$value) = each($_FILES['images']['name'])) { if(!empty($value)) { $filename = $value; $filename=str_replace(" ","_",$filename);// Add _ inplace of blank space in file name, you can remove this line $add = "img/$filename"; copy($_FILES['images']['tmp_name'][$key], $add); chmod("$add",0777); } } } ?> <table border='0' width='400' cellspacing='0' cellpadding='0' align=center> <form method=post action="" enctype='multipart/form-data'> <?php $max_no_img=4; for($i=1; $i<=$max_no_img; $i++){ echo "<tr><td>Images $i</td><td> <input type=file name='images[]'></td></tr>"; } ?> <tr><td colspan=2 align=center><input name="Submit" type=submit value='Add Image'></td></tr> </form> </table>
Saturday, March 3, 2018
jQuery PHP checkbox values to PHP $_POST variables
jQuery PHP checkbox values to PHP $_POST variables
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>jQuery PHP checkbox values to PHP $_POST variables</title> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css"> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script type = "text/javascript"> $(document).ready(function () { $('#submitBtn').click (function() { var selected = new Array(); $("input:checkbox[name=programming]:checked").each(function() { selected.push($(this).val()); }); var selectedString = selected.join(","); $.post("ajaxcheckboxvalue.php", {selected: selected }, function(data){ $('.result').html(data); }); }); }); </script> </head> <body> <div class="container"> <div class="row"> <div class="col-md-6 offset-md-3"> <h1>jQuery PHP checkbox values to PHP $_POST variables</h1> <div class="card" style="margin:50px 0"> <div class="card-header">Checkbox Animation</div> <ul class="list-group list-group-flush"> <li class="list-group-item"> Bootstrap Checkbox Default <label class="checkbox"> <input type="checkbox" name="programming" value="Bootstrap Checkbox Default"/> <span class="default"></span> </label> </li> <li class="list-group-item"> Bootstrap Checkbox Primary <label class="checkbox"> <input type="checkbox" name="programming" value="Bootstrap Checkbox Primary"/> <span class="primary"></span> </label> </li> <li class="list-group-item"> Bootstrap Checkbox Success <label class="checkbox"> <input type="checkbox" name="programming" value="Bootstrap Checkbox Success"/> <span class="success"></span> </label> </li> <li class="list-group-item"> Bootstrap Checkbox Info <label class="checkbox"> <input type="checkbox" name="programming" value="Bootstrap Checkbox Info"/> <span class="info"></span> </label> </li> <li class="list-group-item"> Bootstrap Checkbox Warning <label class="checkbox"> <input type="checkbox" name="programming" value="Bootstrap Checkbox Warning"/> <span class="warning"></span> </label> </li> <li class="list-group-item"> Bootstrap Checkbox Danger <label class="checkbox"> <input type="checkbox" name="programming" value="Bootstrap Checkbox Danger"/> <span class="danger"></span> </label> </li> </ul> <div class = "result" style="padding:10px;"></div> <button type="button" id = "submitBtn" class="btn btn-outline-success slideright" style="margin:10px;">Submit</button> </div> </div> </div> </div> <style> @keyframes check {0% {height: 0;width: 0;} 25% {height: 0;width: 10px;} 50% {height: 20px;width: 10px;} } .checkbox{background-color:#fff;display:inline-block;height:28px;margin:0 .25em;width:28px;border-radius:4px;border:1px solid #ccc;float:right} .checkbox span{display:block;height:28px;position:relative;width:28px;padding:0} .checkbox span:after{-moz-transform:scaleX(-1) rotate(135deg);-ms-transform:scaleX(-1) rotate(135deg);-webkit-transform:scaleX(-1) rotate(135deg);transform:scaleX(-1) rotate(135deg);-moz-transform-origin:left top;-ms-transform-origin:left top;-webkit-transform-origin:left top;transform-origin:left top;border-right:4px solid #fff;border-top:4px solid #fff;content:'';display:block;height:20px;left:3px;position:absolute;top:15px;width:10px} .checkbox span:hover:after{border-color:#999} .checkbox input{display:none} .checkbox input:checked + span:after{-webkit-animation:check .8s;-moz-animation:check .8s;-o-animation:check .8s;animation:check .8s;border-color:#555} .checkbox input:checked + .default:after{border-color:#444} .checkbox input:checked + .primary:after{border-color:#2196F3} .checkbox input:checked + .success:after{border-color:#8bc34a} .checkbox input:checked + .info:after{border-color:#3de0f5} .checkbox input:checked + .warning:after{border-color:#FFC107} .checkbox input:checked + .danger:after{border-color:#f44336} </style> </body> </html>
//ajaxcheckboxvalue.php <?php $selected = $_POST['selected']; foreach ($selected as $value) { echo $value . " <br/>"; } ?>
Saturday, February 24, 2018
Create a PHP jQuery ajax and MySQLi LIKE Search
Create a PHP jQuery ajax and MySQLi LIKE Search
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Create a PHP jQuery ajax and MySQLi LIKE Search</title> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.0/jquery.min.js"></script> <script type="text/javascript"> $(function() { $(".search_button").click(function() { // getting the value that user typed var searchString = $("#search_box").val(); // forming the queryString var data = 'search='+ searchString; // if searchString is not empty if(searchString) { // ajax call $.ajax({ type: "POST", url: "do_search.php", data: data, beforeSend: function(html) { // this happens before actual call $("#results").html(''); $("#searchresults").show(); $(".word").html(searchString); }, success: function(html){ // this happens after we get results $("#results").show(); $("#results").append(html); } }); } return false; }); }); </script> </head> <body> <div id="container"> <div style="margin:20px auto; text-align: center;"> <form method="post" action=""> <input type="text" name="search" id="search_box" class='search_box'/> <input type="submit" value="Search" class="search_button" /><br /> </form> </div> <div> <div id="searchresults">Search results :</div> <div id="results" class="update"></div> </div> </div> <style> body{ font-family:Arial, Helvetica, sans-serif; } #container { margin: 0 auto; width: 600px; } #search_box { padding:4px; border:solid 1px #666666; width:300px; height:30px; font-size:18px;-moz-border-radius: 6px;-webkit-border-radius: 6px; } .search_button { border:#000000 solid 1px; padding: 6px; color:#000; font-weight:bold; font-size:16px;-moz-border-radius: 6px;-webkit-border-radius: 6px; } #searchresults { text-align:left; margin-top:20px; display:none; font-family:Arial, Helvetica, sans-serif; font-size:16px; color:#000; } #newspaper-b { font-family: lucida sans unicode,lucida grande,Sans-Serif; font-size: 12px; width: 480px; text-align: left; border-collapse: collapse; border: 1px solid #69c; margin: 20px; } .tr, tr { border-bottom: 1px solid #ddd; } #newspaper-b th { font-weight: 400; font-size: 14px; color: #039; padding: 15px 10px 10px; } #newspaper-b tbody { background: #e8edff; } #newspaper-b td { color: #669; border-top: 1px dashed #fff; padding: 10px; } #newspaper-b tbody tr:hover td{color:#339;background:#d0dafd} .found { font-weight: bold; font-style: italic; color: #ff0000; } </style> </body> </html>
//do_search.php <table id="newspaper-b"> <thead> <tr> <th scope="col">Name</th> <th scope="col">Category</th> <th scope="col">Price</th> <th scope="col">Discount</th> </tr> </thead> <tbody> <?php include("dbcon.php"); //if we got something through $_POST if (isset($_POST['search'])) { // never trust what user wrote! We must ALWAYS sanitize user input $word = mysql_real_escape_string($_POST['search']); $word = htmlentities($word); // build your search query to the database $sql = "SELECT * FROM product WHERE name LIKE '%" . $word . "%' ORDER BY pid LIMIT 10"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { $end_result = ''; while($row = mysqli_fetch_assoc($result)) { $rs_name = $row["name"]; $category = $row["category"]; $price = $row["price"]; $discount = $row["discount"]; $bold = '<span class="found">' . $word . '</span>'; $end_result .= '<tr><td>'. str_ireplace($word, $bold, $rs_name).'</td><td>'.$category.'</td><td>'.$price.'</td><td>'.$discount.'</td></tr>'; } echo $end_result; }else { echo "0 results"; } } ?> </tbody> </table>
//dbcon.php <?php $conn = new mysqli('localhost','root','','testingdb'); if ($conn->connect_error) { die('Error : ('. $conn->connect_errno .') '. $conn->connect_error); } ?>
Sunday, January 14, 2018
How to Add Widgets to WordPress Theme’s Footer

1. Register the footer widget area
Open the functions.php file from the WordPress Theme Editor and search for the following line of code:
register_sidebar
Add the following block of code just below the other sidebar registration code
function twentysixteen_widgets_init() {
register_sidebar( array(
'name' => __( 'Sidebar', 'twentysixteen' ),
'id' => 'sidebar-1',
'description' => __( 'Add widgets here to appear in your sidebar.', 'twentysixteen' ),
'before_widget' => '<section id="%1$s" class="widget %2$s">',
'after_widget' => '</section>',
'before_title' => '<h2 class="widget-title">',
'after_title' => '</h2>',
) );
register_sidebar( array(
'name' => __( 'Content Bottom 1', 'twentysixteen' ),
'id' => 'sidebar-2',
'description' => __( 'Appears at the bottom of the content on posts and pages.', 'twentysixteen' ),
'before_widget' => '<section id="%1$s" class="widget %2$s">',
'after_widget' => '</section>',
'before_title' => '<h2 class="widget-title">',
'after_title' => '</h2>',
) );
register_sidebar( array(
'name' => __( 'Content Bottom 2', 'twentysixteen' ),
'id' => 'sidebar-3',
'description' => __( 'Appears at the bottom of the content on posts and pages.', 'twentysixteen' ),
'before_widget' => '<section id="%1$s" class="widget %2$s">',
'after_widget' => '</section>',
'before_title' => '<h2 class="widget-title">',
'after_title' => '</h2>',
) );
register_sidebar( array(
'name' => __( 'Content Bottom 3', 'twentysixteen' ),
'id' => 'sidebar-4',
'description' => __( 'Appears at the bottom of the content on posts and pages.', 'twentysixteen' ),
'before_widget' => '<section id="%1$s" class="widget %2$s">',
'after_widget' => '</section>',
'before_title' => '<h2 class="widget-title">',
'after_title' => '</h2>',
) );
}
add_action( 'widgets_init', 'twentysixteen_widgets_init' );
2. Show the footer widget area in your theme
Open your footer.php file and insert the following block of code where you want to show the footer widgets (this will show the 3 footer widget areas):
<div id="footer-sidebar" class="secondary"> <div id="footer-sidebar1"> <?php if(is_active_sidebar('sidebar-2')){ dynamic_sidebar('sidebar-2'); } ?> </div> <div id="footer-sidebar2"> <?php if(is_active_sidebar('sidebar-3')){ dynamic_sidebar('sidebar-3'); } ?> </div> <div id="footer-sidebar3"> <?php if(is_active_sidebar('sidebar-4')){ dynamic_sidebar('sidebar-4'); } ?> </div> </div>
wp_nav_menu change sub-menu class name?

from <ul class="sub-menu"> to <ul class="dropdown-menu">
You can use WordPress preg_replace filter (in your theme functions.php file) example:
function new_submenu_class($menu) { $menu = preg_replace('/ class="sub-menu"/',' class="dropdown-menu" ',$menu); return $menu; } add_filter('wp_nav_menu','new_submenu_class');
Subscribe to:
Posts (Atom)