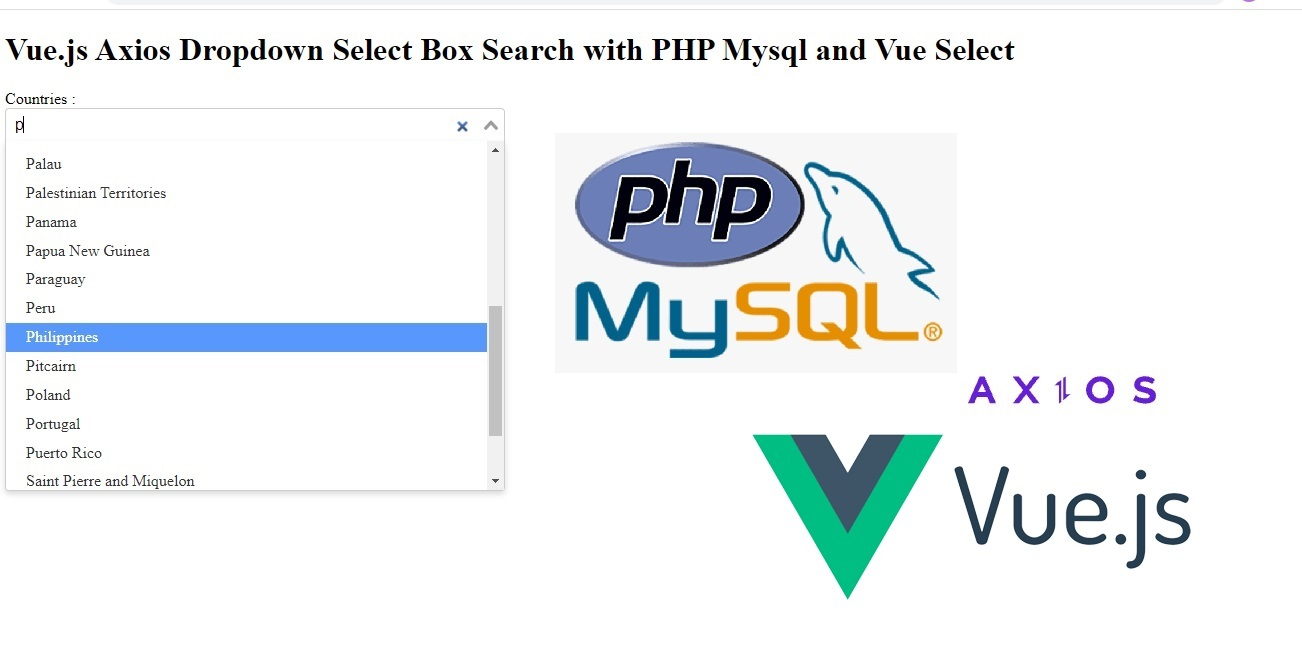
https://vue-select.org/
Vue Select is a feature rich select/dropdown/typeahead component. It provides a default template that fits most use cases for a filterable select dropdown.
https://vue-select.org/guide/install.html
axios
https://github.com/axios/axios
Using jsDelivr CDN: https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js
VueJS
https://vuejs.org/v2/guide/installation.html
CDN : https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js
CREATE TABLE `country` (
`id` int(6) NOT NULL,
`country` varchar(250) NOT NULL DEFAULT ''
) ENGINE=MyISAM DEFAULT CHARSET=latin1;
//index.html <!DOCTYPE html> <html> <head> <title>Vue.js Axios Dropdown Select Box Search with PHP Mysql</title> <link rel="stylesheet" href="https://unpkg.com/vue-select@3.0.0/dist/vue-select.css"> </head> <body> <div id="app"> <p><h1>Vue.js Axios Dropdown Select Box Search with PHP Mysql and Vue Select </h1></p> <div style="width:500px;"> <p>Countries : <v-select v-model="country" label="name" :reduce="country => country.id" :options="country_options" @search="fetchOptions" @input="selectedOption" > </v-select> </p> <p>Selected Value : <span v-text="country"></span></p> </div> </div> <script src="https://unpkg.com/vue@latest"></script> <script src="https://unpkg.com/vue-select@latest"></script> <script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script> <script> Vue.component('v-select', VueSelect.VueSelect) new Vue({ el: '#app', data: { country: 0, country_options: [] }, methods: { fetchOptions: function(search){ var el = this; // AJAX request axios.get('select.php', { params: { search: search, } }) .then(function (response) { // Update options el.country_options = response.data; }); }, selectedOption: function(value){ console.log("value : " + value); } } }) </script> </body> </html>select.php
//select.php <?php $host = "localhost"; $user = "root"; $password = ""; $dbname = "testingdb"; $con = mysqli_connect($host, $user, $password,$dbname); // Check connection if (!$con) { die("Connection failed: " . mysqli_connect_error()); } $search = ""; if(isset($_GET['search'])){ $search = mysqli_real_escape_string($con,$_GET['search']); } $query = 'SELECT * FROM country where country like "%'.$search.'%" '; $result = mysqli_query($con,$query); $response_arr = array(); while($datarow = mysqli_fetch_assoc($result)){ $id = $datarow['id']; $name = $datarow['country']; $response_arr[] = array( "id"=> $id, "name" => $name ); } echo json_encode($response_arr); exit;