<!DOCTYPE html> <html lang="en"> <head> <title>Notification Box, Alert Boxes using Jquery CSS</title> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.2/jquery.min.js"></script> <style> div.alert-message { display: block; padding: 13px 12px 12px; font-weight: bold; font-size: 14px; color: white; background-color: #2ba6cb; border: 1px solid rgba(0, 0, 0, 0.1); margin-bottom: 12px; -webkit-border-radius: 3px; -moz-border-radius: 3px; -ms-border-radius: 3px; -o-border-radius: 3px; border-radius: 3px; text-shadow: 0 -1px rgba(0, 0, 0, 0.3); position: relative; } div.alert-message .box-icon { display: block; float: left; background-image: url('images/icon.png'); width: 30px; height: 25px; margin-top: -2px; background-position: -8px -8px; } div.alert-message p { margin: 0px; } div.alert-message.success { background-color: #5da423; color: #fff; text-shadow: 0 -1px rgba(0, 0, 0, 0.3); } div.alert-message.success .box-icon { background-position: -48px -8px; } div.alert-message.warning { background-color: #e3b000; color: #fff; text-shadow: 0 -1px rgba(0, 0, 0, 0.3); } div.alert-message.warning .box-icon { background-position: -88px -8px; } div.alert-message.error { background-color: #c60f13; color: #fff; text-shadow: 0 -1px rgba(0, 0, 0, 0.3); } div.alert-message.error .box-icon { background-position: -128px -8px; } div.alert-message a.close { color: #333; position: absolute; right: 4px; top: -1px; font-size: 17px; opacity: 0.2; padding: 4px; } div.alert-message a.close:hover, div.alert-box a.close:focus { opacity: 0.4; } </style> <!-- JavaScript Test Zone --> <script type="text/javascript"> $(function(){ $(".alert-message").delegate("a.close", "click", function(event) { event.preventDefault(); $(this).closest(".alert-message").fadeOut(function(event){ $(this).remove(); }); }); }); </script> </head> <body> <div class="panels"> <div class="panel" id="panel-1"> <div class="alert-message info"> <div class="box-icon"></div> <p>This is an info box<a href="" class="close">×</a> </div> <div class="alert-message success"> <div class="box-icon"></div> <p>This is a success box<a href="" class="close">×</a> </div> <div class="alert-message warning"> <div class="box-icon"></div> <p>This is a warning box<a href="" class="close">×</a> </div> <div class="alert-message error"> <div class="box-icon"></div> <p>This is an alert box<a href="" class="close">×</a> </div> </div> </div> </body> </html>
article
Saturday, August 13, 2016
Notification Box, Alert Boxes using Jquery CSS
Notification Box, Alert Boxes using Jquery CSS
Friday, August 12, 2016
CodeIgniter - Upload an Image
CodeIgniter - Upload an Image
Copy the following code and store it at application/view/Upload_form.php.
Copy the following code and store it at application/view/Upload_form.php.
<html> <head> <title>Upload Form</title> </head> <body> <?php echo $error;?> <?php echo form_open_multipart('upload/do_upload');?> <form action = "" method = ""> <input type = "file" name = "userfile" size = "20" /> <br /><br /> <input type = "submit" value = "upload" /> </form> </body> </html>
Copy the code given below and store it at application/view/upload_success.php
<html> <head> <title>Upload Image</title> </head> <body> <h3>Upload an Image Success!</h3> </body> </html>
Copy the code given below and store it at application/controllers/upload.php. Create "uploads" folder at the root of CodeIgniter i.e. at the parent directory of application folder.
<?php class Upload extends CI_Controller { public function __construct() { parent::__construct(); $this->load->helper(array('form', 'url')); } public function index() { $this->load->view('upload_form', array('error' => ' ' )); } public function do_upload() { $config['upload_path'] = './uploads/'; $config['allowed_types'] = 'gif|jpg|png'; $config['max_size'] = 100; $config['max_width'] = 1024; $config['max_height'] = 768; $this->load->library('upload', $config); if ( ! $this->upload->do_upload('userfile')) { $error = array('error' => $this->upload->display_errors()); $this->load->view('upload_form', $error); } else { $data = array('upload_data' => $this->upload->data()); $this->load->view('upload_success', $data); } } } ?>
Make the following change in the route file in application/config/routes.php and add the following line at the end of file.
$route['upload'] = 'Upload';
Now let us execute this example by visiting the following URL in the browser. Replace the yoursite.com with your URL.
http://yoursite.com/index.php/upload
Download http://bit.ly/2XgSkMN
Django - Template System
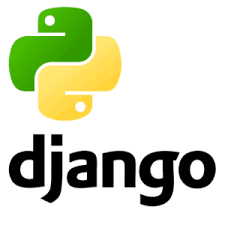
Django makes it possible to separate python and HTML, the python goes in views and HTML goes in templates.
The Render Function
This function takes three parameters −
Request − The initial request.
The path to the template − This is the path relative to the TEMPLATE_DIRS option in the project settings.py variables.
Dictionary of parameters − A dictionary that contains all variables needed in the template. This variable can be created or you can use locals() to pass all local variable declared in the view.
Displaying Variables
A variable looks like this: {{variable}}. The template replaces the variable by the variable sent by the view in the third parameter of the render function.
hello.html
Hello World!!!Today is {{today}}Then our view will change to −
def hello(request): from django.shortcuts import render from django.http import HttpResponse from datetime import datetime def index(request): return render(request, "index.html", {}) def hello(request): myDate = datetime.now() #formatedDate = myDate.strftime("%Y-%m-%d %H:%M:%S") #today = myDate.strftime("%A") today = myDate.strftime("%a") daysOfWeek = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun'] return render(request, "hello.html", {"today" : today, "days_of_week" : daysOfWeek}) #return render(request, "hello.html", {"today" : today})We will now get the following output after accessing the URL/myapp/hello −
Hello World!!!
Today is Sept. 11, 2016
Tag if
Just like in Python you can use if, else and elif in your template −
<html> <body> Hello World!!!<p>Today is {{today}}</p> We are {% if today == 'Sun' %} the Sunday day of month. {% elif today == 30 %} the last day of month. {% else %} I don't know. {%endif%} </body> </html>In this new template, depending on the date of the day, the template will render a certain value.
Tag for
Just like 'if', we have the 'for' tag, that works exactly like in Python. Let's change our hello view to transmit a list to our template −
def hello(request): today = datetime.datetime.now().date() daysOfWeek = ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun'] return render(request, "hello.html", {"today" : today, "days_of_week" : daysOfWeek})The template to display that list using {{ for }} −
<html> <body> Hello World!!!<p>Today is {{today}}</p> We are {% if today.day == 1 %} the first day of month. {% elif today == 30 %} the last day of month. {% else %} I don't know. {%endif%} <p> {% for day in days_of_week %} {{day}} </p> {% endfor %} </body> </html>And we should get something like −
Hello World!!!
Today is Sept. 11, 2015
We are I don't know.
Mon
Tue
Wed
Thu
Fri
Sat
Sun
Block and Extend Tags
A template system cannot be complete without template inheritance. Meaning when you are designing your templates, you should have a main template with holes that the child's template will fill according to his own need, like a page might need a special css for the selected tab.
Let’s change the hello.html template to inherit from a main_template.html.
main_template.html
<html> <head> <title> {% block title %}Page Title{% endblock %} </title> </head> <body> {% block content %} Body content {% endblock %} </body> </html>hello.html
{% extends "main_template.html" %} {% block title %}My Hello Page{% endblock %} {% block content %} Hello World!!!Today is {{today}}
We are {% if today.day == 1 %} the first day of month. {% elif today == 30 %} the last day of month. {% else %} I don't know. {%endif%} {% for day in days_of_week %} {{day}}
{% endfor %} {% endblock %}
Cool Notification Messages With CSS3 & jQuery
Cool Notification Messages With CSS3 & jQuery
<!DOCTYPE html> <html> <head> <title>Cool notification messages with CSS3 & Jquery</title> <script src="http://code.jquery.com/jquery-latest.min.js"></script> <script> var myMessages = ['info','warning','error','success']; // define the messages types function hideAllMessages() { var messagesHeights = new Array(); // this array will store height for each for (i=0; i<myMessages.length; i++) { messagesHeights[i] = $('.' + myMessages[i]).outerHeight(); $('.' + myMessages[i]).css('top', -messagesHeights[i]); //move element outside viewport } } function showMessage(type) { $('.'+ type +'-trigger').click(function(){ hideAllMessages(); $('.'+type).animate({top:"0"}, 500); }); } $(document).ready(function(){ // Initially, hide them all hideAllMessages(); // Show message for(var i=0;i<myMessages.length;i++) { showMessage(myMessages[i]); } // When message is clicked, hide it $('.message').click(function(){ $(this).animate({top: -$(this).outerHeight()}, 500); }); }); </script> <style> body { margin: 0; padding: 0; font: 12px Arial, Helvetica, sans-serif; background: #f1f1f1; } .message { -webkit-background-size: 40px 40px; -moz-background-size: 40px 40px; background-size: 40px 40px; background-image: -webkit-gradient(linear, left top, right bottom, color-stop(.25, rgba(255, 255, 255, .05)), color-stop(.25, transparent), color-stop(.5, transparent), color-stop(.5, rgba(255, 255, 255, .05)), color-stop(.75, rgba(255, 255, 255, .05)), color-stop(.75, transparent), to(transparent)); background-image: -webkit-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: -moz-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: -ms-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: -o-linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); background-image: linear-gradient(135deg, rgba(255, 255, 255, .05) 25%, transparent 25%, transparent 50%, rgba(255, 255, 255, .05) 50%, rgba(255, 255, 255, .05) 75%, transparent 75%, transparent); -moz-box-shadow: inset 0 -1px 0 rgba(255,255,255,.4); -webkit-box-shadow: inset 0 -1px 0 rgba(255,255,255,.4); box-shadow: inset 0 -1px 0 rgba(255,255,255,.4); width: 100%; border: 1px solid; color: #fff; padding: 15px; position: fixed; _position: absolute; text-shadow: 0 1px 0 rgba(0,0,0,.5); -webkit-animation: animate-bg 5s linear infinite; -moz-animation: animate-bg 5s linear infinite; } .info { background-color: #4ea5cd; border-color: #3b8eb5; } .error { background-color: #de4343; border-color: #c43d3d; } .warning { background-color: #eaaf51; border-color: #d99a36; } .success { background-color: #61b832; border-color: #55a12c; } .message h3 { margin: 0 0 5px 0; } .message p { margin: 0; } @-webkit-keyframes animate-bg { from { background-position: 0 0; } to { background-position: -80px 0; } } @-moz-keyframes animate-bg { from { background-position: 0 0; } to { background-position: -80px 0; } } #trigger-list { text-align: center; margin: 100px 0; padding: 0; } #trigger-list li { display: inline-block; *display: inline; zoom: 1; } #trigger-list .trigger { display: inline-block; background: #ddd; border: 1px solid #777; padding: 10px 20px; margin: 0 5px; font: bold 12px Arial, Helvetica; text-decoration: none; color: #333; -moz-border-radius: 3px; -webkit-border-radius: 3px; border-radius: 3px; } #trigger-list .trigger:hover { background: #f5f5f5; } .centered { text-align: center; } .twitter-follow-button { position: relative; top: 7px; } </style> </head> <body> <div class="info message"> <h3>FYI, something just happened!</h3> <p>This is just an info notification message.</p> </div> <div class="error message"> <h3>Ups, an error ocurred</h3> <p>This is just an error notification message.</p> </div> <div class="warning message"> <h3>Wait, I must warn you!</h3> <p>This is just a warning notification message.</p> </div> <div class="success message"> <h3>Congrats, you did it!</h3> <p>This is just a success notification message.</p> </div> <ul id="trigger-list"> <li><a href="#" class="trigger info-trigger">Info</a></li> <li><a href="#" class="trigger error-trigger">Error</a></li> <li><a href="#" class="trigger warning-trigger">Warning</a></li> <li><a href="#" class="trigger success-trigger">Success</a></li> </ul> </body> </html>
Subscribe to:
Posts (Atom)